Create3DCurve.cpp
119 built = (built && createPortIn<dvecarr3E, Create3DCurve>(this, &mimmo::Create3DCurve::setRawPoints, M_COORDS, true,1));
120 built = (built && createPortIn<dvecarr3E, Create3DCurve>(this, &mimmo::Create3DCurve::setRawVectorField, M_DISPLS));
121 built = (built && createPortIn<dvector1D, Create3DCurve>(this, &mimmo::Create3DCurve::setRawScalarField, M_DATAFIELD));
123 built = (built && createPortIn<dmpvecarr3E*, Create3DCurve>(this, &mimmo::Create3DCurve::setRawPoints, M_VECTORFIELD, true,1));
124 built = (built && createPortIn<dmpvecarr3E*, Create3DCurve>(this, &mimmo::Create3DCurve::setRawVectorField, M_VECTORFIELD2));
125 built = (built && createPortIn<dmpvector1D*, Create3DCurve>(this, &mimmo::Create3DCurve::setRawScalarField, M_SCALARFIELD));
127 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, Create3DCurve>(this, &mimmo::Create3DCurve::getGeometry, M_GEOM));
128 built = (built && createPortOut<dmpvector1D*, Create3DCurve>(this, &mimmo::Create3DCurve::getScalarField, M_SCALARFIELD));
129 built = (built && createPortOut<dmpvecarr3E*, Create3DCurve>(this, &mimmo::Create3DCurve::getVectorField, M_VECTORFIELD));
276 void Create3DCurve::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
400 m_geometry->addConnectedCell(livector1D(tuple.second.begin(),tuple.second.end()), bitpit::ElementType::LINE, 0, tuple.first, 0);
430 Create3DCurve::fillPreliminaryStructure(dmpvecarr3E & points, std::unordered_map<long, std::array<long,2> > &connectivity){
467 Create3DCurve::refineObject(dmpvecarr3E & points, dmpvector1D & scalarf, dmpvecarr3E & vectorf, std::unordered_map<long, std::array<long,2> > &connectivity, int fCells){
488 std::priority_queue<std::pair<double,long>, std::vector<std::pair<double,long>>, greatDist > pQue(mycomp, values);
504 scalarf.insert(idV, 0.5*(scalarf[connectivity[pp.second][1]] + scalarf[connectivity[pp.second][0]]));
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setRawVectorField(dvecarr3E rawVectorField)
Definition: Create3DCurve.cpp:191
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void plotOptionalResults()
Definition: Create3DCurve.cpp:325
void setRawPoints(dvecarr3E rawPoints)
Definition: Create3DCurve.cpp:167
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setRawScalarField(dvector1D rawScalarField)
Definition: Create3DCurve.cpp:215
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
mimmo::MimmoPiercedVector< double > dmpvector1D
Definition: MimmoPiercedVector.hpp:125
@ POINT
void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Create3DCurve.cpp:311
Create3DCurve & operator=(Create3DCurve other)
Definition: Create3DCurve.cpp:90
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Create3DCurve.cpp:276
mimmo::MimmoPiercedVector< darray3E > dmpvecarr3E
Definition: MimmoPiercedVector.hpp:129
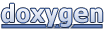