Distribute a raw list of points on a target 3D surface. More...
#include <CreateSeedsOnSurface.hpp>
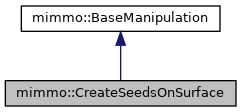
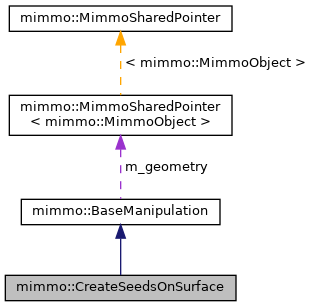
Protected Member Functions | |
void | checkField () |
bool | checkTriangulation () |
MimmoSharedPointer< MimmoObject > | createTriangulation () |
void | normalizeField () |
virtual void | plotOptionalResults () |
void | swap (CreateSeedsOnSurface &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Distribute a raw list of points on a target 3D surface.
Class/BaseManipulation Object to create a raw set of 3D points lying on a 3D surface.
MPI version will treat this raw list as shared among the ranks (every rank will retain exactly the same list)
Three type of engines to compute point positions are available:
- CSeedSurf::RANDOM : sows points randomly on surface, trying to displace them at maximum euclidean distance;
- CSeedSurf::CARTESIANGRID evaluate points by projection of a volumetric cartesian grid of surface and decimating the list up the desired value of points, trying to displace them at maximum euclidean distance possible.
- CSeedSurf::LEVELSET : starting from an initial seed, sows points around it, trying to displace them at maximum geodesic distance possible on the surface; BEWARE this option is not available in MPI version with np processors > 1.
Default engine is CARTESIANGRID.
A Sensitivity field, defined on the target 3D surface vertices can be linked, to drive the seeding procedure, i.e. to displace points in the most sensible location according to the field.
Ports available in CreateSeedsOnSurface Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | setSeed | (MC_ARRAY3, MD_FLOAT) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_FILTER | setSensitivityField | (MC_SCALAR, MD_MPVECFLOAT_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | getPoints | (MC_VECARR3, MD_FLOAT) |
M_VALUEI | getRandomSignature | (MC_SCALAR, MD_INT) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.CreateSeedsOnSurface
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: plot optional result during object execution;
- OutputPlot: set path to store optional result.
Proper of the class:
- NPoints: total points to distribute;
- Engine: type of distribution engine 0:Random,2:CartesianGrid,1:Levelset(NOT AVAILABLE IN MPI np>1 VERSION);
- Seed: initial seed point coordinates (space separated);
- MassCenterAsSeed: boolean (0/1), if true use geometry mass center as seed;
- RandomFixed: boolean(0/1), if active it fixes distribution pattern when 0:RANDOM engine is selected, otherwise leave it changing as it likes;
- RandomSignature: specify unsigned integer seed when RandomFixed is active. This will reproduce the same random distribution each run;
- Examples
- manipulators_example_00008.cpp, and utils_example_00001.cpp.
Definition at line 111 of file CreateSeedsOnSurface.hpp.
Constructor & Destructor Documentation
◆ CreateSeedsOnSurface() [1/3]
mimmo::CreateSeedsOnSurface::CreateSeedsOnSurface | ( | ) |
! raw list of interpolated sensitivities on shared points
Constructor
Definition at line 41 of file CreateSeedsOnSurface.cpp.
◆ CreateSeedsOnSurface() [2/3]
mimmo::CreateSeedsOnSurface::CreateSeedsOnSurface | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 57 of file CreateSeedsOnSurface.cpp.
◆ ~CreateSeedsOnSurface()
|
virtual |
Destructor;
Definition at line 82 of file CreateSeedsOnSurface.cpp.
◆ CreateSeedsOnSurface() [3/3]
mimmo::CreateSeedsOnSurface::CreateSeedsOnSurface | ( | const CreateSeedsOnSurface & | other | ) |
Copy constructor. Retain only meaningful inputs
- Parameters
-
[in] other class to copy from
Definition at line 88 of file CreateSeedsOnSurface.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 1389 of file CreateSeedsOnSurface.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 135 of file CreateSeedsOnSurface.cpp.
◆ checkField()
|
protected |
Check your current point data sensitivity field associated to linked geometry. Do nothing if class linked geometry is a null pointer or empty. If field geometry is not linked or empty or if field geometry its uncoherent with class linked geometry or not referred to MPVLocation point or if the field itself does not carry any value, create a default unitary field. If the field is still coherent but miss values on some geometry nodes, complete it assigning zero value on missing ids. If no exception occurs, leave the field as it is.
Definition at line 1506 of file CreateSeedsOnSurface.cpp.
◆ checkTriangulation()
|
protected |
- Returns
- true if the linked geometry is a homogeneous triangular 3D surface, false otherwise
Definition at line 1560 of file CreateSeedsOnSurface.cpp.
◆ clear()
void mimmo::CreateSeedsOnSurface::clear | ( | ) |
Clear input contents of the class
Definition at line 348 of file CreateSeedsOnSurface.cpp.
◆ createTriangulation()
|
protected |
- Returns
- a homogeneous triangulated and indipendent clone of the current target geometry linked to the class. set also this new geometry to member sensitivity triangulated
Definition at line 1576 of file CreateSeedsOnSurface.cpp.
◆ execute()
|
virtual |
Execution command of the class. Wrapper to CreateSeedsOnSurface::solve method
Implements mimmo::BaseManipulation.
Definition at line 366 of file CreateSeedsOnSurface.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 1463 of file CreateSeedsOnSurface.cpp.
◆ getEngine()
int mimmo::CreateSeedsOnSurface::getEngine | ( | ) |
Return engine type for point distribution calculation.
- Returns
- engine type
Definition at line 184 of file CreateSeedsOnSurface.cpp.
◆ getEngineENUM()
CSeedSurf mimmo::CreateSeedsOnSurface::getEngineENUM | ( | ) |
Return CSeedSurf engine type for point distribution calculation.
- Returns
- engine type
- Examples
- utils_example_00001.cpp.
Definition at line 174 of file CreateSeedsOnSurface.cpp.
◆ getMinDistance()
double mimmo::CreateSeedsOnSurface::getMinDistance | ( | ) |
Return minimum absolute distance during the point distribution calculation.
- Returns
- minimum distance between points
Definition at line 215 of file CreateSeedsOnSurface.cpp.
◆ getNPoints()
int mimmo::CreateSeedsOnSurface::getNPoints | ( | ) |
Return number of points to be distributed over 3D surface.
- Returns
- number of points
Definition at line 156 of file CreateSeedsOnSurface.cpp.
◆ getPoints()
dvecarr3E mimmo::CreateSeedsOnSurface::getPoints | ( | ) |
Return list of points distributed over 3D surface, after class execution.
- Returns
- list of points
Definition at line 165 of file CreateSeedsOnSurface.cpp.
◆ getRandomSignature()
long mimmo::CreateSeedsOnSurface::getRandomSignature | ( | ) |
Return the signature of the current random distribution of points on target surface, whenever is fixed or not, for result replication. See setRandomFixed method. This option will only make sense if a CSeedSurf::RANDOM engine is employed. Otherwise it is ignored.
- Returns
- signature.
- Examples
- utils_example_00001.cpp.
Definition at line 237 of file CreateSeedsOnSurface.cpp.
◆ getSeed()
darray3E mimmo::CreateSeedsOnSurface::getSeed | ( | ) |
Return seed point used for calculation. If geometry baricenter is used as initial seed (see setMassCenterAsSeed), it can be correctly visualized after the execution of the object.
- Returns
- seed point
Definition at line 195 of file CreateSeedsOnSurface.cpp.
◆ isRandomFixed()
bool mimmo::CreateSeedsOnSurface::isRandomFixed | ( | ) |
Return true, if the option to fix Random distribution through signature is active. False otherwise. This option will only make sense if a CSeedSurf::RANDOM engine is employed.
- Returns
- boolean
Definition at line 226 of file CreateSeedsOnSurface.cpp.
◆ isSeedMassCenter()
bool mimmo::CreateSeedsOnSurface::isSeedMassCenter | ( | ) |
Return true, if the option to use geometry mass center as initial seed is activated. (see setMassCenterAsSeed method documentation).
- Returns
- mass center flag
Definition at line 205 of file CreateSeedsOnSurface.cpp.
◆ normalizeField()
|
protected |
Normalize your current point data sensitivity field associated to linked geometry. It is assumed at this point that the field is fully coeherent, as result of checkField method check.
Definition at line 1534 of file CreateSeedsOnSurface.cpp.
◆ operator=()
CreateSeedsOnSurface & mimmo::CreateSeedsOnSurface::operator= | ( | CreateSeedsOnSurface | other | ) |
Assignment operator
- Parameters
-
[in] other class to copy from
Definition at line 105 of file CreateSeedsOnSurface.cpp.
◆ plotCloud()
void mimmo::CreateSeedsOnSurface::plotCloud | ( | std::string | dir, |
std::string | file, | ||
int | counter, | ||
bool | binary | ||
) |
Plot point distribution as *.vtu Cloud point.
- Parameters
-
[in] dir folder path [in] file file name without tag [in] counter identifier integer for the file [in] binary flag to write ASCII-false, binary-true
Definition at line 825 of file CreateSeedsOnSurface.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot Optional results of the class, that is the point cloud distribution on surface as a list of xyz data points w/ identifier mark and as a point cloud in *.vtu format
Reimplemented from mimmo::BaseManipulation.
Definition at line 376 of file CreateSeedsOnSurface.cpp.
◆ setEngine()
void mimmo::CreateSeedsOnSurface::setEngine | ( | int | eng | ) |
Set the engine type for point distribution evaluation
- Parameters
-
[in] eng engine type passed as integer. See CSeedSurf enum.
- Examples
- utils_example_00001.cpp.
Definition at line 264 of file CreateSeedsOnSurface.cpp.
◆ setEngineENUM()
void mimmo::CreateSeedsOnSurface::setEngineENUM | ( | CSeedSurf | eng | ) |
Set the engine type for point distribution evaluation
- Parameters
-
[in] eng engine type passed as enum CSeedSurf
- Examples
- manipulators_example_00008.cpp.
Definition at line 255 of file CreateSeedsOnSurface.cpp.
◆ setGeometry()
void mimmo::CreateSeedsOnSurface::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Set Geometry, check if its search bvtree is built, if not, build it. Point Cloud geometries, or pure Volume meshes are currently not supported by this block. Reimplemented from BaseManipulation::setGeometry()
- Parameters
-
[in] geo pointer to target geometry
- Examples
- utils_example_00001.cpp.
Definition at line 297 of file CreateSeedsOnSurface.cpp.
◆ setMassCenterAsSeed()
void mimmo::CreateSeedsOnSurface::setMassCenterAsSeed | ( | bool | flag | ) |
Set the starting seed point for points distribution on surface evaluation as the mass center of the surface itself. Any seed passed with setSeed method will be ignored if this option is active.
- Parameters
-
[in] flag true activate the option, false deactivate it.
- Examples
- manipulators_example_00008.cpp.
Definition at line 286 of file CreateSeedsOnSurface.cpp.
◆ setNPoints()
void mimmo::CreateSeedsOnSurface::setNPoints | ( | int | val | ) |
Set the number of points to be distributed.
- Parameters
-
[in] val number of total points
- Examples
- manipulators_example_00008.cpp, and utils_example_00001.cpp.
Definition at line 246 of file CreateSeedsOnSurface.cpp.
◆ setRandomFixed()
void mimmo::CreateSeedsOnSurface::setRandomFixed | ( | bool | fix | ) |
Activate a fixed random distribution through signature specification (see setRandomSignature). Same signature will be able to reproduce the exact random distribution in multiple runs. It is possible to get the current signature after each random execution using the getRandomSignature method. This option will only make sense if a CSeedSurf::RANDOM engine is employed. Otherwise it is ignored.
- Parameters
-
[in] fix if true activate the options, false deactivate it.
- Examples
- manipulators_example_00008.cpp, and utils_example_00001.cpp.
Definition at line 316 of file CreateSeedsOnSurface.cpp.
◆ setRandomSignature()
void mimmo::CreateSeedsOnSurface::setRandomSignature | ( | uint32_t | signature | ) |
Set the signature (each integer >= 0) of your fixed random distribution (activated by setRandomFixed). Same signature will be able to reproduce the exact random distribution in multiple runs. This option will only make sense if a CSeedSurf::RANDOM engine is employed. Otherwise it is ignored.
- Parameters
-
[in] signature unsigned integer number
- Examples
- manipulators_example_00008.cpp, and utils_example_00001.cpp.
Definition at line 328 of file CreateSeedsOnSurface.cpp.
◆ setSeed()
void mimmo::CreateSeedsOnSurface::setSeed | ( | darray3E | seed | ) |
Set the starting seed point for points distribution on surface evaluation. The assigned seed is ignored if the geometry baricenter is set as initial seed (see setMassCenterAsSeed).
- Parameters
-
[in] seed starting 3D point in the neighborhood of the surface
- Examples
- utils_example_00001.cpp.
Definition at line 276 of file CreateSeedsOnSurface.cpp.
◆ setSensitivityMap()
void mimmo::CreateSeedsOnSurface::setSensitivityMap | ( | dmpvector1D * | field | ) |
Set a sensitivity scalar field, referred to the target surface geometry linked, to drive points seeding onto the most sensitive part of the geometry. The sensitivity field MUST be defined on geometry vertices.
- Parameters
-
[in] field sensitivity
- Examples
- utils_example_00001.cpp.
Definition at line 339 of file CreateSeedsOnSurface.cpp.
◆ solve()
void mimmo::CreateSeedsOnSurface::solve | ( | bool | debug = false | ) |
Apply command of the class;
- Parameters
-
[in] debug flag to activate logs of solver execution
Definition at line 386 of file CreateSeedsOnSurface.cpp.
◆ swap()
|
protectednoexcept |
Swap function.
- Parameters
-
[in] x object to be swapped
Definition at line 114 of file CreateSeedsOnSurface.cpp.
The documentation for this class was generated from the following files:
- src/utils/CreateSeedsOnSurface.hpp
- src/utils/CreateSeedsOnSurface.cpp
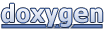