CreateSeedsOnSurface.hpp
187 void solveEikonal(double g, double s, bitpit::PatchKernel &tri,std::unordered_map<long,long> & invConn, dmpvector1D & field);
188 double updateEikonal(double g, double s, long tVert,long tCell,bitpit::PatchKernel &tri, std::unordered_map<long int, short int> &flag, dmpvector1D & field);
MimmoSharedPointer< MimmoObject > createTriangulation()
Definition: CreateSeedsOnSurface.cpp:1576
bool isSeedMassCenter()
Definition: CreateSeedsOnSurface.cpp:205
bool checkTriangulation()
Definition: CreateSeedsOnSurface.cpp:1560
CSeedSurf
Enum class for engine choice to set up initial points on a 3D surface.
Definition: CreateSeedsOnSurface.hpp:39
@ RANDOM
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: CreateSeedsOnSurface.cpp:1463
@ LEVELSET
void setRandomSignature(uint32_t signature)
Definition: CreateSeedsOnSurface.cpp:328
bool isRandomFixed()
Definition: CreateSeedsOnSurface.cpp:226
void setRandomFixed(bool fix)
Definition: CreateSeedsOnSurface.cpp:316
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: CreateSeedsOnSurface.cpp:1389
long getRandomSignature()
Definition: CreateSeedsOnSurface.cpp:237
void setSensitivityMap(dmpvector1D *field)
Definition: CreateSeedsOnSurface.cpp:339
void swap(CreateSeedsOnSurface &x) noexcept
Definition: CreateSeedsOnSurface.cpp:114
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void solve(bool debug=false)
Definition: CreateSeedsOnSurface.cpp:386
double getMinDistance()
Definition: CreateSeedsOnSurface.cpp:215
Distribute a raw list of points on a target 3D surface.
Definition: CreateSeedsOnSurface.hpp:111
@ CARTESIANGRID
CSeedSurf getEngineENUM()
Definition: CreateSeedsOnSurface.cpp:174
virtual ~CreateSeedsOnSurface()
Definition: CreateSeedsOnSurface.cpp:82
void normalizeField()
Definition: CreateSeedsOnSurface.cpp:1534
void setEngineENUM(CSeedSurf)
Definition: CreateSeedsOnSurface.cpp:255
void plotCloud(std::string dir, std::string file, int counter, bool binary)
Definition: CreateSeedsOnSurface.cpp:825
CreateSeedsOnSurface & operator=(CreateSeedsOnSurface other)
Definition: CreateSeedsOnSurface.cpp:105
void setGeometry(MimmoSharedPointer< MimmoObject >)
Definition: CreateSeedsOnSurface.cpp:297
virtual void plotOptionalResults()
Definition: CreateSeedsOnSurface.cpp:376
CreateSeedsOnSurface()
Definition: CreateSeedsOnSurface.cpp:41
void setMassCenterAsSeed(bool)
Definition: CreateSeedsOnSurface.cpp:286
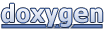