GenericOutput is the class that write generic data in a file output. More...
#include <GenericOutput.hpp>
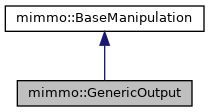
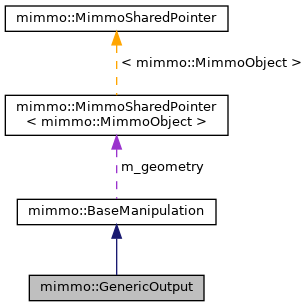
Public Member Functions | |
GenericOutput (const bitpit::Config::Section &rootXML) | |
GenericOutput (const GenericOutput &other) | |
GenericOutput (std::string dir="./", std::string filename="output.txt", bool csv=false) | |
~GenericOutput () | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clearInput () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
template<typename T > | |
T * | getInput () |
GenericOutput & | operator= (GenericOutput other) |
void | setCSV (bool csv) |
void | setFilename (std::string filename) |
template<typename T > | |
void | setInput (T *data) |
template<typename T > | |
void | setInput (T data) |
void | setWriteDir (std::string dir) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | swap (GenericOutput &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
GenericOutput is the class that write generic data in a file output.
GenericOutput is derived from BaseManipulation class. GenericOutput can write the output in a data file (unformatted/csv). When writing, the GenericOutput object recognizes the type of data and it adapts the writing method in function of the input port used to build link (pin) with an other object.
On distributed archs, only the 0 rank procs is deputed to writing. Since the class does not hold data structure that can be unambigously recollected once partitioned, it is supposed that all procs hold the same identical data.
Ports available in GenericOutput Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | setResult | (MC_VECARR3, MD_FLOAT) |
M_DISPLS | setResult | (MC_VECARR3, MD_FLOAT) |
M_DATAFIELD | setResult | (MC_VECTOR, MD_FLOAT) |
M_POINT | setResult | (MC_ARRAY3, MD_FLOAT) |
M_SPAN | setResult | (MC_ARRAY3, MD_FLOAT) |
M_DIMENSION | setResult | (MC_ARRAY3, MD_INT) |
M_VALUED | setResult | (MC_SCALAR, MD_FLOAT) |
M_VALUEI | setResult | (MC_SCALAR, MD_INT) |
M_VALUEB | setResult | (MC_SCALAR, MD_BOOL) |
M_DEG | setResult | (MC_ARRAY3, MD_INT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.GenericOutput
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- Filename: name of file to write data;
- WriteDir: name of directory to write data;
- CSV: true if write in csv format;
- Examples
- genericinput_example_00001.cpp, manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 109 of file GenericOutput.hpp.
Constructor & Destructor Documentation
◆ GenericOutput() [1/3]
mimmo::GenericOutput::GenericOutput | ( | std::string | dir = "./" , |
std::string | filename = "output.txt" , |
||
bool | csv = false |
||
) |
Default constructor of GenericOutput
- Parameters
-
[in] dir Directory of the output file (default value = "./"). [in] filename Name of the output file (default value = "output.txt"). [in] csv True if the output file is a csv format file (default value false).
Definition at line 34 of file GenericOutput.cpp.
◆ GenericOutput() [2/3]
mimmo::GenericOutput::GenericOutput | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 46 of file GenericOutput.cpp.
◆ ~GenericOutput()
mimmo::GenericOutput::~GenericOutput | ( | ) |
Default destructor of GenericOutput.
Definition at line 67 of file GenericOutput.cpp.
◆ GenericOutput() [3/3]
mimmo::GenericOutput::GenericOutput | ( | const GenericOutput & | other | ) |
Copy constructor of GenericOutput. m_input member is not copied.
Definition at line 72 of file GenericOutput.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 174 of file GenericOutput.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 103 of file GenericOutput.cpp.
◆ clearInput()
void mimmo::GenericOutput::clearInput | ( | ) |
It clear the input member of the object
Definition at line 152 of file GenericOutput.cpp.
◆ execute()
|
virtual |
Execution command. It does nothing, the real execution of the object happens in setInput.
Implements mimmo::BaseManipulation.
Definition at line 161 of file GenericOutput.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 212 of file GenericOutput.cpp.
◆ getInput()
T * mimmo::GenericOutput::getInput |
It gets the input member of the object.
- Returns
- Pointer to data stored in the input member.
Definition at line 207 of file GenericOutput.tpp.
◆ operator=()
GenericOutput & mimmo::GenericOutput::operator= | ( | GenericOutput | other | ) |
Assignment operator
Definition at line 81 of file GenericOutput.cpp.
◆ setCSV()
void mimmo::GenericOutput::setCSV | ( | bool | csv | ) |
It sets if the output file has to be written in csv format.
- Parameters
-
[in] csv Write the output file in csv format.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 143 of file GenericOutput.cpp.
◆ setFilename()
void mimmo::GenericOutput::setFilename | ( | std::string | filename | ) |
It sets the name of the output file.
- Parameters
-
[in] filename Name of the output file.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 134 of file GenericOutput.cpp.
◆ setInput() [1/2]
void mimmo::GenericOutput::setInput | ( | T * | data | ) |
Overloaded function of base class setInput. It sets the input/result and write on file at the same time.
- Parameters
-
[in] data Pointer to data to be written and to be used to set the input/result.
Definition at line 170 of file GenericOutput.tpp.
◆ setInput() [2/2]
void mimmo::GenericOutput::setInput | ( | T | data | ) |
Overloaded function of base class setInput. It sets the input/result and write on file at the same time.
- Parameters
-
[in] data Data to be written and to be used to set the input/result.
Definition at line 197 of file GenericOutput.tpp.
◆ setWriteDir()
void mimmo::GenericOutput::setWriteDir | ( | std::string | dir | ) |
It sets the name of the directory to write the output file.
- Parameters
-
[in] dir Name of the directory of the output file.
Definition at line 125 of file GenericOutput.cpp.
◆ swap()
|
protectednoexcept |
The documentation for this class was generated from the following files:
- src/iogeneric/GenericOutput.hpp
- src/iogeneric/GenericOutput.cpp
- src/iogeneric/GenericOutput.tpp
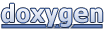