GenericOutput.hpp
215 GenericOutputMPVData(std::string dir = "./", std::string filename = "output.txt", bool csv = false);
249 void collectDataFromAllProcs(MimmoPiercedVector<T> & locdata, MimmoPiercedVector<T> * dataglobal);
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:174
GenericOutputMPVData is the class that write a generic data to file output as mimmo::MimmoPiercedVect...
Definition: GenericOutput.hpp:203
void setFilename(std::string filename)
Definition: GenericOutput.cpp:326
void swap(GenericOutputMPVData &x) noexcept
Definition: GenericOutput.cpp:289
void setInput(MimmoPiercedVector< T > *data)
Definition: GenericOutput.tpp:227
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:375
GenericOutput & operator=(GenericOutput other)
Definition: GenericOutput.cpp:81
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:423
void setFilename(std::string filename)
Definition: GenericOutput.cpp:134
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
MimmoPiercedVector is the basic data container for mimmo library.
Definition: MimmoPiercedVector.hpp:60
GenericOutput is the class that write generic data in a file output.
Definition: GenericOutput.hpp:109
GenericOutputMPVData(std::string dir="./", std::string filename="output.txt", bool csv=false)
Definition: GenericOutput.cpp:230
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:212
std::fstream & ofstreamcsvend(std::fstream &in, const T &x)
Definition: GenericOutput.tpp:49
~GenericOutputMPVData()
Definition: GenericOutput.cpp:265
void setWriteDir(std::string dir)
Definition: GenericOutput.cpp:317
std::fstream & ofstreamcsv(std::fstream &in, const T &x)
Definition: GenericOutput.tpp:36
GenericOutputMPVData & operator=(GenericOutputMPVData other)
Definition: GenericOutput.cpp:280
MimmoPiercedVector< T > * getInput()
Definition: GenericOutput.tpp:349
GenericOutput(std::string dir="./", std::string filename="output.txt", bool csv=false)
Definition: GenericOutput.cpp:34
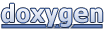