GenericOutput.cpp
106 built = (built && createPortIn<dvecarr3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<dvecarr3E>, M_COORDS, true, 1));
107 built = (built && createPortIn<dvecarr3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<dvecarr3E>, M_DISPLS, true, 1));
108 built = (built && createPortIn<dvector1D, GenericOutput>(this, &mimmo::GenericOutput::setInput<dvector1D>, M_DATAFIELD, true, 1));
109 built = (built && createPortIn<darray3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<darray3E>, M_POINT, true, 1));
110 built = (built && createPortIn<darray3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<darray3E>, M_SPAN, true, 1));
111 built = (built && createPortIn<iarray3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<iarray3E>, M_DIMENSION, true, 1));
112 built = (built && createPortIn<double, GenericOutput>(this, &mimmo::GenericOutput::setInput<double>, M_VALUED, true, 1));
113 built = (built && createPortIn<int, GenericOutput>(this, &mimmo::GenericOutput::setInput<int>, M_VALUEI, true, 1));
114 built = (built && createPortIn<bool, GenericOutput>(this, &mimmo::GenericOutput::setInput<bool>, M_VALUEB, true, 1));
115 built = (built && createPortIn<iarray3E, GenericOutput>(this, &mimmo::GenericOutput::setInput<iarray3E>, M_DEG, true, 1));
270 GenericOutputMPVData::GenericOutputMPVData(const GenericOutputMPVData & other):BaseManipulation(other){
306 built = (built && createPortIn<dmpvector1D*, GenericOutputMPVData>(this, &mimmo::GenericOutputMPVData::setInput<double>, M_SCALARFIELD));
307 built = (built && createPortIn<dmpvecarr3E*, GenericOutputMPVData>(this, &mimmo::GenericOutputMPVData::setInput<darray3E>, M_VECTORFIELD));
375 GenericOutputMPVData::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:174
GenericOutputMPVData is the class that write a generic data to file output as mimmo::MimmoPiercedVect...
Definition: GenericOutput.hpp:203
void setFilename(std::string filename)
Definition: GenericOutput.cpp:326
void swap(GenericOutputMPVData &x) noexcept
Definition: GenericOutput.cpp:289
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:375
GenericOutput & operator=(GenericOutput other)
Definition: GenericOutput.cpp:81
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:423
void setFilename(std::string filename)
Definition: GenericOutput.cpp:134
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
GenericOutput is the class that write generic data in a file output.
Definition: GenericOutput.hpp:109
GenericOutputMPVData(std::string dir="./", std::string filename="output.txt", bool csv=false)
Definition: GenericOutput.cpp:230
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericOutput.cpp:212
~GenericOutputMPVData()
Definition: GenericOutput.cpp:265
void setWriteDir(std::string dir)
Definition: GenericOutput.cpp:317
GenericOutputMPVData & operator=(GenericOutputMPVData other)
Definition: GenericOutput.cpp:280
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
GenericOutput(std::string dir="./", std::string filename="output.txt", bool csv=false)
Definition: GenericOutput.cpp:34
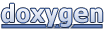