BendGeometry applies custom bending deformations along axis-directions of a target geometry. More...
#include <BendGeometry.hpp>
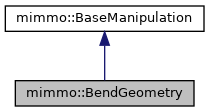
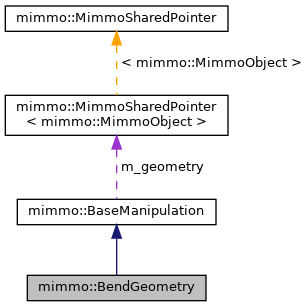
Protected Member Functions | |
void | checkFilter () |
void | swap (BendGeometry &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Static Protected Member Functions | |
static darray3E | matmul (const darray3E &vec, const dmatrix33E &mat) |
static darray3E | matmul (const dmatrix33E &mat, const darray3E &vec) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
BendGeometry applies custom bending deformations along axis-directions of a target geometry.
Bending deformation is defined as a vector field of displacements along a certain axis (0-x,1-y or 2-z) and modelized by a set of custom polynomial functions of N degree. Each displacement coordinate, for each axis can be influenced by its own bending (9 possible bending functions). In particular, for each displacement component \(i\in[0,2]\) the bending is expressed as:
\( S_i = \sum_{j,k}( a_{ijk} x_{j}^k ) \)
where \(a_{ijk}\) is the polynomial coefficient of term of degree \(k\in[0,N]\), related to axis \(j\in[0,2]\) and applied to the \(i\)-th displacement coordinate.
Ports available in BendGeometry Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_FILTER | setFilter | (MC_SCALAR, MD_MPVECFLOAT_) |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXES | setRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_BMATRIX | setDegree | (MC_ARR3ARR3, MD_INT) |
M_BCOEFFS | setCoeffs | (MC_SCALAR, MD_MATRIXCOEFF_) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | getDisplacements | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited form BaseManipulation:
- ClassName : name of the class as
mimmo.BendGeometry
; - Priority : uint marking priority of class execution in multichain frame;
- Apply: boolean 0/1 activate apply deformation result on target geometry directly in execution;
Proper of the class:
- DegreesMatrix(3x3): degrees \(d_{ij}\) of each polynomial function referred to a displacement component \(i\in[0,2]\) and modulating it along axis \(j\in[0,2]\). \(d_{ij} = 0\) marks a constant function. Written in XML as:
<DegreesMatrix>
<xDispl> 1 0 0 </xDispl> (linear x-displacement distribution in x bending direction)
<yDispl> 2 0 0 </yDispl> (quadratic y-displacement distribution in x bending direction)
<zDispl> 0 3 0 </zDispl> (cubic z-displacement in y bending direction)
</DegreesMatrix>
- PolyCoefficients: coefficients of each 9 bending polynomial functions. Writing following the enumeration \(n = 3i + j\), where i is the displacement component and j the axis of bending. For example, n=7 corresponds to i=2, j=1, reflecting in a z-component displacement distribution in y-axis bending direction. Please note the number of coefficients for a ij-bending function is equal to the function degree \(d_{ij}\) + 1 (DegreesMatrix), ordered as \(c_0 + c_1x +c_2x^2+...+c_nx^n\). If a polynomial function is not specified, it will be treated as 0 constant function. Written in XML as :
<PolyCoefficients>
<Poly0> 1.0 1.5 </Poly0>
<Poly3> -0.1 0.2 -0.01 </Poly3>
<Poly7> 1.5 0.0 0.1 0.2 </Poly7>
...
</PolyCoefficients>
- Origin: 3D point marking the origin of reference system (default {0,0,0});
- RefSystem: define a bending custom reference system of axes. Written in XML as:
\<RefSystem>
<axis0> 1.0 0.0 0.0 </axis0>
<axis1> 0.0 1.0 0.0 </axis1>
<axis2> 0.0 0.0 1.0 </axis2>
</RefSystem>
Geometry has to be mandatorily passed through port.
- Examples
- manipulators_example_00001.cpp.
Definition at line 120 of file BendGeometry.hpp.
Constructor & Destructor Documentation
◆ BendGeometry() [1/3]
mimmo::BendGeometry::BendGeometry | ( | ) |
Default constructor of BendGeometry
Definition at line 32 of file BendGeometry.cpp.
◆ BendGeometry() [2/3]
mimmo::BendGeometry::BendGeometry | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 45 of file BendGeometry.cpp.
◆ ~BendGeometry()
mimmo::BendGeometry::~BendGeometry | ( | ) |
Default destructor of BendGeometry
Definition at line 66 of file BendGeometry.cpp.
◆ BendGeometry() [3/3]
mimmo::BendGeometry::BendGeometry | ( | const BendGeometry & | other | ) |
Copy constructor of BendGeometry. Resulting displacements are not copied.
Definition at line 71 of file BendGeometry.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 409 of file BendGeometry.cpp.
◆ apply()
|
virtual |
Directly apply deformation field to target geometry.
Reimplemented from mimmo::BaseManipulation.
Definition at line 302 of file BendGeometry.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 107 of file BendGeometry.cpp.
◆ checkFilter()
|
protected |
Check if the filter is related to the target geometry. If not create a unitary filter field.
Definition at line 311 of file BendGeometry.cpp.
◆ execute()
|
virtual |
Execution command. It computes the displacements on geometry nodes with the bending polynomial set. The displacements are stored in local m_displ variable.
Implements mimmo::BaseManipulation.
Definition at line 247 of file BendGeometry.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 500 of file BendGeometry.cpp.
◆ getCoeffs()
dmat33Evec * mimmo::BendGeometry::getCoeffs | ( | ) |
It gets the matrix of coefficients of the polynomial functions. coeffs[i][j] = \({a_{ij0},a_{ij1},a_{ij2},...,a_{ijN}}\)
- Returns
- polynomials coefficents matrix.
Definition at line 150 of file BendGeometry.cpp.
◆ getDegree()
umatrix33E mimmo::BendGeometry::getDegree | ( | ) |
It gets the degrees of polynomial law for each component of displacements of degrees of freedom.
- Returns
- Degrees of polynomial laws (degree[i][j] = degree of displacement function si = f(xj)).
Definition at line 140 of file BendGeometry.cpp.
◆ getDisplacements()
dmpvecarr3E * mimmo::BendGeometry::getDisplacements | ( | ) |
- Returns
- bending displacements computed on geometry nodes
Definition at line 158 of file BendGeometry.cpp.
◆ getOrigin()
darray3E mimmo::BendGeometry::getOrigin | ( | ) |
- Returns
- current origin of reference system
Definition at line 124 of file BendGeometry.cpp.
◆ getRefSystem()
dmatrix33E mimmo::BendGeometry::getRefSystem | ( | ) |
- Returns
- current reference system axes
Definition at line 132 of file BendGeometry.cpp.
◆ matmul() [1/2]
|
staticprotected |
Matrix M-vector V multiplication V*M (V row dot M columns).
- Parameters
-
[in] vec vector V with 3 elements [in] mat matrix M 3x3 square
- Returns
- 3 element vector result of multiplication
Definition at line 371 of file BendGeometry.cpp.
◆ matmul() [2/2]
|
staticprotected |
Matrix M-vector V multiplication M*V (M rows dot V column).
- Parameters
-
[in] vec vector V with 3 elements [in] mat matrix M 3x3 square
- Returns
- 3 element vector result of multiplication
Definition at line 390 of file BendGeometry.cpp.
◆ operator=()
BendGeometry & mimmo::BendGeometry::operator= | ( | BendGeometry | other | ) |
Assignment operator.
Definition at line 83 of file BendGeometry.cpp.
◆ setCoeffs() [1/2]
void mimmo::BendGeometry::setCoeffs | ( | dmat33Evec * | coeffs | ) |
It sets the matrix of coefficients of the polynomial functions. Singular entry ij of the matrix is a vector coeffs[i][j] = \({a_{ij0},a_{ij1},a_{ij2},...,a_{ijN}}\)
- Parameters
-
[in] coeffs pointer to matrix of coefficients
- Examples
- manipulators_example_00001.cpp.
Definition at line 194 of file BendGeometry.cpp.
◆ setCoeffs() [2/2]
void mimmo::BendGeometry::setCoeffs | ( | int | i, |
int | j, | ||
dvector1D | coeffs | ||
) |
It sets the coefficients of polynomial \(P_{ij}\)
- Parameters
-
[in] i component of displacement vector [in] j coordinate of the axis [in] coeffs vector of polynomial coefficients coeffs[i][j] = \({a_{ij0},a_{ij1},a_{ij2},...,a_{ijN}}\)
Definition at line 206 of file BendGeometry.cpp.
◆ setDegree() [1/2]
void mimmo::BendGeometry::setDegree | ( | int | i, |
int | j, | ||
uint32_t | degree | ||
) |
It sets the degree \(d_{ij}\) of polynomial \(P_{ij}\).
- Parameters
-
[in] i component of displacement vector. [in] j coordinate of the axis [in] degree \(d_{ij}\)
Definition at line 182 of file BendGeometry.cpp.
◆ setDegree() [2/2]
void mimmo::BendGeometry::setDegree | ( | umatrix33E | degree | ) |
It sets the degrees matrix (3x3) of each polynomial law.
- Parameters
-
[in] degree matrix of degrees \(d_{ij}\)
- Examples
- manipulators_example_00001.cpp.
Definition at line 167 of file BendGeometry.cpp.
◆ setFilter()
void mimmo::BendGeometry::setFilter | ( | dmpvector1D * | filter | ) |
It sets a filter field to modulate the displacementsof the target geometry nodes.
- Parameters
-
[in] filter field defined on geometry vertices.
Definition at line 237 of file BendGeometry.cpp.
◆ setOrigin()
void mimmo::BendGeometry::setOrigin | ( | darray3E | origin | ) |
It sets the origin of the local reference system.
- Parameters
-
[in] origin of local reference system.
Definition at line 215 of file BendGeometry.cpp.
◆ setRefSystem()
void mimmo::BendGeometry::setRefSystem | ( | dmatrix33E | axes | ) |
It sets a new axes orientation frame for bending s.d.r.
- Parameters
-
[in] axes matrix.
Definition at line 225 of file BendGeometry.cpp.
◆ swap()
|
protectednoexcept |
The documentation for this class was generated from the following files:
- src/manipulators/BendGeometry.hpp
- src/manipulators/BendGeometry.cpp
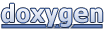