BendGeometry.hpp
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BendGeometry.cpp:409
BendGeometry & operator=(BendGeometry other)
Definition: BendGeometry.cpp:83
BendGeometry applies custom bending deformations along axis-directions of a target geometry.
Definition: BendGeometry.hpp:120
dmpvecarr3E * getDisplacements()
Definition: BendGeometry.cpp:158
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
static darray3E matmul(const darray3E &vec, const dmatrix33E &mat)
Definition: BendGeometry.cpp:371
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BendGeometry.cpp:500
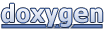