ExtractField is an abstract executable block class for extracting/restricting an input field defined on a mesh X on a target subportion of X. More...
#include <ExtractFields.hpp>
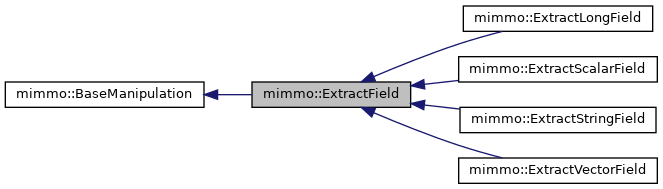
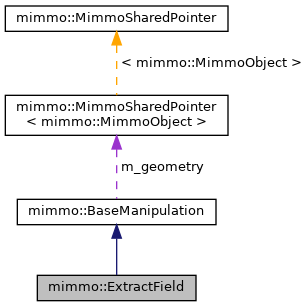
Public Member Functions | |
ExtractField () | |
ExtractField (const ExtractField &other) | |
virtual | ~ExtractField () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clear () |
void | execute () |
virtual bool | extract ()=0 |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
ExtractMode | getMode () |
double | getTolerance () |
ExtractField & | operator= (const ExtractField &other) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setMode (ExtractMode mode) |
void | setMode (int mode) |
void | setTolerance (double tol) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | swap (ExtractField &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
ExtractMode | m_mode |
double | m_tol |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
ExtractField is an abstract executable block class for extracting/restricting an input field defined on a mesh X on a target subportion of X.
ExtractField classes take as inputs:
- a target input field defined on a geometry
- a subportion of the geometry where the input field is defined.
Reference data location (POINT, CELLS or INTERFACES) of the input field is used to extract data on vertices, cells or interfaces of the target geometry. Three methods are available:
- ID = 1 : extraction of data on reference Location with the same IDs between target and input field linked geometry. Return an extracted field referred to the target geometry, with the same data location of the input field.
- PID = 2 : extraction of data on reference Location within common PIDs between target geometry and field linked geometry; Return an extracted field referred to the input field linked geometry, with the same data location of the input field.
- MAPPING = 3 : extraction of data on reference Location identified by a proximity mapping between target geometry and field linked geometry; Return an extracted field referred to the target geometry,with the same data location of the input field.
ExtractField is an abstract class. To use its features take a look to its specializations, here presented as derived class, ExtractScalarField and ExtractVectorField.
Ports available in ExtractField Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.Extract<...>Field
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class.
- OutputPlot: target directory for optional results writing.
Proper of the class:
- ExtractMode: activate extraction mode by ID(1), PID(2) or MAPPING(3);
- Tolerance: tolerance for extraction by patch, meaningful only in Mapping mode.
Geometries and fields have to be mandatorily passed through port.
Definition at line 95 of file ExtractFields.hpp.
Constructor & Destructor Documentation
◆ ExtractField() [1/2]
mimmo::ExtractField::ExtractField | ( | ) |
Default constructor of ExtractField.
Definition at line 31 of file ExtractFields.cpp.
◆ ~ExtractField()
|
virtual |
Default destructor of ExtractField.
Definition at line 39 of file ExtractFields.cpp.
◆ ExtractField() [2/2]
mimmo::ExtractField::ExtractField | ( | const ExtractField & | other | ) |
Copy Constructor.
- Parameters
-
[in] other class of type ExtractField
Definition at line 47 of file ExtractFields.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 157 of file ExtractFields.cpp.
◆ buildPorts()
|
virtual |
Build the ports of the class;
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::ExtractStringField, mimmo::ExtractLongField, mimmo::ExtractVectorField, and mimmo::ExtractScalarField.
Definition at line 78 of file ExtractFields.cpp.
◆ clear()
void mimmo::ExtractField::clear | ( | ) |
Clear all stuffs in your class
Definition at line 134 of file ExtractFields.cpp.
◆ execute()
|
virtual |
Execution command. Extract a field over a target geometry from an input field.
Implements mimmo::BaseManipulation.
Definition at line 142 of file ExtractFields.cpp.
◆ extract()
|
pure virtual |
Pure virtual method
Implemented in mimmo::ExtractStringField, mimmo::ExtractLongField, mimmo::ExtractVectorField, and mimmo::ExtractScalarField.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 194 of file ExtractFields.cpp.
◆ getMode()
ExtractMode mimmo::ExtractField::getMode | ( | ) |
Get current extraction mode set on the class.
- Returns
- type of extraction in enum ExtractMode
Definition at line 126 of file ExtractFields.cpp.
◆ getTolerance()
double mimmo::ExtractField::getTolerance | ( | ) |
Get tolerance for extraction by patch actually set in the class.
- Returns
- tolerance
Definition at line 117 of file ExtractFields.cpp.
◆ operator=()
ExtractField & mimmo::ExtractField::operator= | ( | const ExtractField & | other | ) |
Assignement operator of ExtractField.
- Parameters
-
[in] other class of type ExtractField
Definition at line 67 of file ExtractFields.cpp.
◆ setGeometry()
void mimmo::BaseManipulation::setGeometry |
It sets the geometry linked by the manipulator object.
- Parameters
-
[in] geometry Pointer to geometry to be deformed by the manipulator object.
Definition at line 433 of file BaseManipulation.cpp.
◆ setMode() [1/2]
void mimmo::ExtractField::setMode | ( | ExtractMode | mode | ) |
Set mode of extraction.
- Parameters
-
[in] mode Extraction mode
- Examples
- geohandlers_example_00003.cpp, and iocgns_example_00001.cpp.
Definition at line 89 of file ExtractFields.cpp.
◆ setMode() [2/2]
void mimmo::ExtractField::setMode | ( | int | mode | ) |
Set mode of extraction.
- Parameters
-
[in] mode Extraction mode
Definition at line 98 of file ExtractFields.cpp.
◆ setTolerance()
void mimmo::ExtractField::setTolerance | ( | double | tol | ) |
Set tolerance for extraction by patch.
- Parameters
-
[in] tol Tolerance
- Examples
- geohandlers_example_00003.cpp.
Definition at line 108 of file ExtractFields.cpp.
◆ swap()
|
protectednoexcept |
Swap function of ExtractField
- Parameters
-
[in] x object to be swapped.
Definition at line 56 of file ExtractFields.cpp.
Member Data Documentation
◆ m_mode
|
protected |
Extraction mode.
Definition at line 97 of file ExtractFields.hpp.
◆ m_tol
|
protected |
Tolerance for extraction by patch.
Definition at line 98 of file ExtractFields.hpp.
The documentation for this class was generated from the following files:
- src/geohandlers/ExtractFields.hpp
- src/geohandlers/ExtractFields.cpp
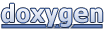