ExtractFields.hpp
void setField(dmpvecarr3E *field)
Definition: ExtractVectorField.cpp:131
ExtractMode
Modes available to extract fields.See class ExtractField documentation.
Definition: ExtractFields.hpp:34
ExtractStringField()
Definition: ExtractStringField.cpp:33
void swap(ExtractLongField &x) noexcept
Definition: ExtractLongField.cpp:86
ExtractScalarField is specialized derived class of ExtractField to extract a scalar field of doubles.
Definition: ExtractFields.hpp:180
MimmoPiercedVector< std::string > getOriginalField()
Definition: ExtractStringField.cpp:123
ExtractScalarField()
Definition: ExtractScalarField.cpp:33
virtual ~ExtractLongField()
Definition: ExtractLongField.cpp:59
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:194
virtual ~ExtractVectorField()
Definition: ExtractVectorField.cpp:59
ExtractLongField & operator=(const ExtractLongField &other)
Definition: ExtractLongField.cpp:75
void setField(MimmoPiercedVector< long > *field)
Definition: ExtractLongField.cpp:132
dmpvecarr3E getOriginalField()
Definition: ExtractVectorField.cpp:122
ExtractScalarField & operator=(const ExtractScalarField &other)
Definition: ExtractScalarField.cpp:75
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
ExtractField is an abstract executable block class for extracting/restricting an input field defined ...
Definition: ExtractFields.hpp:95
void swap(ExtractScalarField &x) noexcept
Definition: ExtractScalarField.cpp:86
ExtractVectorField & operator=(const ExtractVectorField &other)
Definition: ExtractVectorField.cpp:74
void plotOptionalResults()
Definition: ExtractLongField.cpp:151
void plotOptionalResults()
Definition: ExtractScalarField.cpp:151
void setField(MimmoPiercedVector< std::string > *field)
Definition: ExtractStringField.cpp:132
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
dmpvecarr3E * getExtractedField()
Definition: ExtractVectorField.cpp:113
ExtractField & operator=(const ExtractField &other)
Definition: ExtractFields.cpp:67
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual ~ExtractScalarField()
Definition: ExtractScalarField.cpp:59
MimmoPiercedVector< long > getOriginalField()
Definition: ExtractLongField.cpp:123
dmpvector1D getOriginalField()
Definition: ExtractScalarField.cpp:123
ExtractStringField & operator=(const ExtractStringField &other)
Definition: ExtractStringField.cpp:75
void setField(dmpvector1D *field)
Definition: ExtractScalarField.cpp:132
void plotOptionalResults()
Definition: ExtractStringField.cpp:151
void swap(ExtractVectorField &x) noexcept
Definition: ExtractVectorField.cpp:85
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:157
MimmoPiercedVector< std::string > * getExtractedField()
Definition: ExtractStringField.cpp:114
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
dmpvector1D * getExtractedField()
Definition: ExtractScalarField.cpp:114
virtual ~ExtractStringField()
Definition: ExtractStringField.cpp:59
void plotOptionalResults()
Definition: ExtractVectorField.cpp:150
MimmoPiercedVector< long > * getExtractedField()
Definition: ExtractLongField.cpp:114
void swap(ExtractStringField &x) noexcept
Definition: ExtractStringField.cpp:86
@ MAPPING
ExtractVectorField()
Definition: ExtractVectorField.cpp:33
virtual bool extract()=0
ExtractStringField is specialized derived class of ExtractField to extract a scalar field of string.
Definition: ExtractFields.hpp:426
ExtractVectorField is specialized derived class of ExtractField to extract a vector field of array<do...
Definition: ExtractFields.hpp:263
ExtractLongField is specialized derived class of ExtractField to extract a scalar field of long.
Definition: ExtractFields.hpp:343
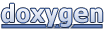