ExtractVectorField.cpp
102 built = (built && createPortIn<dmpvecarr3E*, ExtractVectorField>(this, &mimmo::ExtractVectorField::setField, M_VECTORFIELD, true));
103 built = (built && createPortOut<dmpvecarr3E*, ExtractVectorField>(this, &mimmo::ExtractVectorField::getExtractedField, M_VECTORFIELD));
300 livector1D cellExtracted = mimmo::skdTreeUtils::selectByGlobalPatch(m_field.getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tol);
302 livector1D cellExtracted = mimmo::skdTreeUtils::selectByPatch(m_field.getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tol);
void setField(dmpvecarr3E *field)
Definition: ExtractVectorField.cpp:131
virtual ~ExtractVectorField()
Definition: ExtractVectorField.cpp:59
dmpvecarr3E getOriginalField()
Definition: ExtractVectorField.cpp:122
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
@ CELL
bool checkDataIdsCoherence()
Definition: MimmoPiercedVector.tpp:412
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
ExtractField is an abstract executable block class for extracting/restricting an input field defined ...
Definition: ExtractFields.hpp:95
ExtractVectorField & operator=(const ExtractVectorField &other)
Definition: ExtractVectorField.cpp:74
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
@ INTERFACE
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
dmpvecarr3E * getExtractedField()
Definition: ExtractVectorField.cpp:113
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
ExtractField & operator=(const ExtractField &other)
Definition: ExtractFields.cpp:67
std::vector< long > selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol)
Definition: SkdTreeUtils.cpp:251
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
void swap(ExtractVectorField &x) noexcept
Definition: ExtractVectorField.cpp:85
@ UNDEFINED
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:157
void plotOptionalResults()
Definition: ExtractVectorField.cpp:150
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
@ MAPPING
ExtractVectorField()
Definition: ExtractVectorField.cpp:33
ExtractVectorField is specialized derived class of ExtractField to extract a vector field of array<do...
Definition: ExtractFields.hpp:263
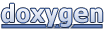