ExtractFields.cpp
80 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ExtractField>(this, &mimmo::ExtractField::setGeometry, M_GEOM, true));
ExtractMode
Modes available to extract fields.See class ExtractField documentation.
Definition: ExtractFields.hpp:34
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:194
ExtractField is an abstract executable block class for extracting/restricting an input field defined ...
Definition: ExtractFields.hpp:95
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
BaseManipulation & operator=(const BaseManipulation &other)
Definition: BaseManipulation.cpp:113
ExtractField & operator=(const ExtractField &other)
Definition: ExtractFields.cpp:67
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:157
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
@ MAPPING
virtual bool extract()=0
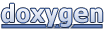