ExtractLongField.cpp
103 built = (built && createPortIn<MimmoPiercedVector<long>*, ExtractLongField>(this, &mimmo::ExtractLongField::setField, M_LONGFIELD, true));
104 built = (built && createPortOut<MimmoPiercedVector<long>*, ExtractLongField>(this, &mimmo::ExtractLongField::getExtractedField, M_LONGFIELD));
301 livector1D cellExtracted = mimmo::skdTreeUtils::selectByGlobalPatch(m_field.getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tol);
303 livector1D cellExtracted = mimmo::skdTreeUtils::selectByPatch(m_field.getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tol);
void swap(ExtractLongField &x) noexcept
Definition: ExtractLongField.cpp:86
virtual ~ExtractLongField()
Definition: ExtractLongField.cpp:59
ExtractLongField & operator=(const ExtractLongField &other)
Definition: ExtractLongField.cpp:75
void setField(MimmoPiercedVector< long > *field)
Definition: ExtractLongField.cpp:132
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
@ CELL
bool checkDataIdsCoherence()
Definition: MimmoPiercedVector.tpp:412
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
ExtractField is an abstract executable block class for extracting/restricting an input field defined ...
Definition: ExtractFields.hpp:95
void plotOptionalResults()
Definition: ExtractLongField.cpp:151
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
@ INTERFACE
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
ExtractField & operator=(const ExtractField &other)
Definition: ExtractFields.cpp:67
MimmoPiercedVector< long > getOriginalField()
Definition: ExtractLongField.cpp:123
std::vector< long > selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol)
Definition: SkdTreeUtils.cpp:251
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
@ UNDEFINED
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ExtractFields.cpp:157
MimmoPiercedVector< long > * getExtractedField()
Definition: ExtractLongField.cpp:114
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
@ MAPPING
ExtractLongField is specialized derived class of ExtractField to extract a scalar field of long.
Definition: ExtractFields.hpp:343
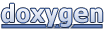