SpecularPoints.cpp
122 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, SpecularPoints>(this,&mimmo::SpecularPoints::setPointCloud, M_GEOM2, true));
123 built = (built && createPortIn<dmpvecarr3E*, SpecularPoints>(this, &mimmo::SpecularPoints::setVectorData, M_VECTORFIELD));
124 built = (built && createPortIn<dmpvector1D*, SpecularPoints>(this, &mimmo::SpecularPoints::setScalarData, M_DATAFIELD));
125 built = (built && createPortIn<darray4E, SpecularPoints>(this, &mimmo::SpecularPoints::setPlane, M_PLANE));
126 built = (built && createPortIn<darray3E, SpecularPoints>(this, &mimmo::SpecularPoints::setOrigin, M_POINT));
127 built = (built && createPortIn<darray3E, SpecularPoints>(this, &mimmo::SpecularPoints::setNormal, M_AXIS));
128 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, SpecularPoints>(this, &mimmo::SpecularPoints::setGeometry, M_GEOM));
130 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, SpecularPoints>(this, &mimmo::SpecularPoints::getMirroredPointCloud, M_GEOM));
131 built = (built && createPortOut<dmpvecarr3E*, SpecularPoints>(this, &mimmo::SpecularPoints::getMirroredVectorData, M_VECTORFIELD));
132 built = (built && createPortOut<dmpvector1D*, SpecularPoints>(this, &mimmo::SpecularPoints::getMirroredScalarData, M_SCALARFIELD));
492 m_cobj->addConnectedCell(std::vector<long>(1, offsetVertLabel), type, 0, offsetCellLabel, m_rank);
bool m_buildKdTree
Definition: ProjPrimitivesOnSurfaces.hpp:76
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SpecularPoints.cpp:554
bool m_workingOnTarget
Definition: ProjPatchOnSurface.hpp:103
livector1D getMirroredLabels()
Definition: SpecularPoints.cpp:195
virtual void plotOptionalResults()
Definition: SpecularPoints.cpp:657
MimmoSharedPointer< MimmoObject > m_patch
Definition: ProjPrimitivesOnSurfaces.hpp:77
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: ProjPrimitivesOnSurfaces.cpp:142
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
dmpvecarr3E * getMirroredVectorData()
Definition: SpecularPoints.cpp:168
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjPatchOnSurface.cpp:205
dmpvecarr3E * getOriginalVectorData()
Definition: SpecularPoints.cpp:150
SpecularPoints is a class that mirrors a point cloud w.r.t. a reference plane, on a target surface ge...
Definition: SpecularPoints.hpp:91
void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjPatchOnSurface.cpp:160
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
dmpvector1D * getOriginalScalarData()
Definition: SpecularPoints.cpp:141
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
void setPointCloud(MimmoSharedPointer< MimmoObject > targetpatch)
Definition: SpecularPoints.cpp:250
@ POINT
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface,...
Definition: ProjPatchOnSurface.hpp:99
dvecarr3E getMirroredRawCoords()
Definition: SpecularPoints.cpp:178
MimmoSharedPointer< MimmoObject > getMirroredPointCloud()
Definition: SpecularPoints.cpp:211
MimmoSharedPointer< MimmoObject > m_cobj
Definition: ProjPatchOnSurface.hpp:102
SpecularPoints & operator=(SpecularPoints other)
Definition: SpecularPoints.cpp:91
void setVectorData(dmpvecarr3E *vdata)
Definition: SpecularPoints.cpp:274
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SpecularPoints.cpp:613
void setScalarData(dmpvector1D *data)
Definition: SpecularPoints.cpp:263
dmpvector1D * getMirroredScalarData()
Definition: SpecularPoints.cpp:159
void swap(ProjPatchOnSurface &x) noexcept
Definition: ProjPatchOnSurface.cpp:98
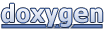