ProjSegmentOnSurface.cpp
83 ProjSegmentOnSurface::ProjSegmentOnSurface(const ProjSegmentOnSurface & other):ProjPrimitivesOnSurfaces(other){
147 void ProjSegmentOnSurface::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
212 void ProjSegmentOnSurface::flushSectionXML(bitpit::Config::Section & slotXML, std::string name){
273 skdTreeUtils::projectPointGlobal(npoints, points.data(), getGeometry()->getSkdTree(), projs.data(), ids.data(), ranks.data(), radius, false);
275 skdTreeUtils::projectPoint(npoints, points.data(), getGeometry()->getSkdTree(), projs.data(), ids.data());
bool m_buildKdTree
Definition: ProjPrimitivesOnSurfaces.hpp:76
void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjSegmentOnSurface.cpp:147
ProjSegmentOnSurface & operator=(ProjSegmentOnSurface other)
Definition: ProjSegmentOnSurface.cpp:91
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
MimmoSharedPointer< MimmoObject > m_patch
Definition: ProjPrimitivesOnSurfaces.hpp:77
virtual ~ProjSegmentOnSurface()
Definition: ProjSegmentOnSurface.cpp:76
bool m_buildSkdTree
Definition: ProjPrimitivesOnSurfaces.hpp:75
void setProjElementTargetNCells(int nC)
Definition: ProjPrimitivesOnSurfaces.cpp:171
ProjSegmentOnSurface()
Definition: ProjSegmentOnSurface.cpp:45
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjSegmentOnSurface.cpp:212
void swap(ProjSegmentOnSurface &x) noexcept
Definition: ProjSegmentOnSurface.cpp:100
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
Class for projecting 1D/2D primitives on a target 3D surface mesh.
Definition: ProjPrimitivesOnSurfaces.hpp:70
void swap(ProjPrimitivesOnSurfaces &x) noexcept
Definition: ProjPrimitivesOnSurfaces.cpp:82
Executable block class capable of projecting an elemental segment on a 3D surface mesh defined by a M...
Definition: ProjSegmentOnSurface.hpp:99
void setBuildSkdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:154
darray3E projectPoint(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *skdtree, double r)
Definition: SkdTreeUtils.cpp:375
void setSegment(darray3E pointA, darray3E pointB)
Definition: ProjSegmentOnSurface.cpp:123
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void setBuildKdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:163
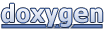