StitchGeometry.cpp
120 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, StitchGeometry>(this, &mimmo::StitchGeometry::addGeometry, M_GEOM, true));
122 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, StitchGeometry>(this, &mimmo::StitchGeometry::getGeometry, M_GEOM));
198 (*m_log)<<"WARNING "<< m_name<<" : stitching partitioned meshes doesn't guarantee unique ids among processors"<<std::endl;
316 void StitchGeometry::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
332 (*m_log)<<"Error in "<<m_name<<" : class Topology parameter mismatch from what is read in XML input"<<std::endl;
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
mimmo::MimmoSharedPointer< MimmoObject > getGeometry()
Definition: StitchGeometry.cpp:140
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
StitchGeometry & operator=(StitchGeometry other)
Definition: StitchGeometry.cpp:93
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void addGeometry(mimmo::MimmoSharedPointer< MimmoObject > geo)
Definition: StitchGeometry.cpp:150
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void plotOptionalResults()
Definition: StitchGeometry.cpp:373
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: StitchGeometry.cpp:316
StitchGeometry is an executable block class capable of stitch multiple MimmoObject geometries of the ...
Definition: StitchGeometry.hpp:72
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: StitchGeometry.cpp:359
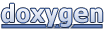