ControlDeformMaxDistance.cpp
64 ControlDeformMaxDistance::ControlDeformMaxDistance(const ControlDeformMaxDistance & other):BaseManipulation(other){
95 built = (built && createPortIn<dmpvecarr3E*, ControlDeformMaxDistance>(this, &mimmo::ControlDeformMaxDistance::setDefField, M_GDISPLS));
96 built = (built && createPortIn<double, ControlDeformMaxDistance>(this, &mimmo::ControlDeformMaxDistance::setLimitDistance, M_VALUED));
97 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ControlDeformMaxDistance>(this, &mimmo::ControlDeformMaxDistance::setGeometry, M_GEOM, true));
99 built = (built && createPortOut<double, ControlDeformMaxDistance>(this, &mimmo::ControlDeformMaxDistance::getViolation, M_VALUED));
100 built = (built && createPortOut<dmpvector1D*, ControlDeformMaxDistance>(this, &mimmo::ControlDeformMaxDistance::getViolationField, M_SCALARFIELD));
200 std::vector<long> mapIDV = geo->getVerticesIds(); //take all internals and ghosts if partitioned mesh.
219 skdTreeUtils::globalDistance(points.size(), points.data(), geo->getSkdTree(), suppCellIds.data(), suppCellRanks.data(), distances.data(), normDef.data(), false);
221 skdTreeUtils::distance(points.size(), points.data(), geo->getSkdTree(), suppCellIds.data(), distances.data(), normDef.data());
260 ControlDeformMaxDistance::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ControlDeformMaxDistance.cpp:260
void buildPorts()
Definition: ControlDeformMaxDistance.cpp:92
void setDefField(dmpvecarr3E *field)
Definition: ControlDeformMaxDistance.cpp:143
double getViolation()
Definition: ControlDeformMaxDistance.cpp:112
void swap(ControlDeformMaxDistance &x) noexcept
Definition: ControlDeformMaxDistance.cpp:81
ControlDeformMaxDistance & operator=(ControlDeformMaxDistance other)
Definition: ControlDeformMaxDistance.cpp:72
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void plotOptionalResults()
Definition: ControlDeformMaxDistance.cpp:300
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
ControlDeformMaxDistance()
Definition: ControlDeformMaxDistance.cpp:32
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: ControlDeformMaxDistance.cpp:166
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
ControlDeformMaxDistance is a class that check a deformation field associated to a MimmoObject surfac...
Definition: ControlDeformMaxDistance.hpp:84
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setLimitDistance(double dist)
Definition: ControlDeformMaxDistance.cpp:156
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
@ POINT
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ControlDeformMaxDistance.cpp:285
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
dmpvector1D * getViolationField()
Definition: ControlDeformMaxDistance.cpp:133
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
double distance(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *tree, long &id, double r)
Definition: SkdTreeUtils.cpp:49
~ControlDeformMaxDistance()
Definition: ControlDeformMaxDistance.cpp:59
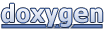