ControlDeformExtSurface.cpp
73 ControlDeformExtSurface::ControlDeformExtSurface(const ControlDeformExtSurface & other):BaseManipulation(other){
109 built = (built && createPortIn<dmpvecarr3E*, ControlDeformExtSurface>(this, &mimmo::ControlDeformExtSurface::setDefField, M_GDISPLS));
110 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ControlDeformExtSurface>(this, &mimmo::ControlDeformExtSurface::setGeometry, M_GEOM, true));
111 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ControlDeformExtSurface>(this, &mimmo::ControlDeformExtSurface::addConstraint, M_GEOM2));
113 built = (built && createPortOut<double, ControlDeformExtSurface>(this, &mimmo::ControlDeformExtSurface::getViolation, M_VALUED));
114 built = (built && createPortOut<dmpvector1D*, ControlDeformExtSurface>(this, &mimmo::ControlDeformExtSurface::getViolationField, M_SCALARFIELD));
194 (*m_log)<<"warning: ControlDeformExtSurface cannot support current geometry. It works only w/ 3D surface."<<std::endl;
220 ControlDeformExtSurface::addConstraint( mimmo::MimmoSharedPointer<mimmo::MimmoObject> constraint){
223 (*m_log)<<"warning: ControlDeformExtSurface cannot support current constraint geometry. It works only w/ 3D surface."<<std::endl;
360 (*m_log)<<"Warning in " + m_name +" : no valid constraint geometries are linked to the class. "<<std::endl;
381 //calculate where the center of the global target is located w.r.t to constraint, to keep up the
386 evaluateSignedDistance(std::vector<darray3E>(1,originalGlobalCenter), localg, searchRadius, distances);
423 ControlDeformExtSurface::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
507 ControlDeformExtSurface::readFileConstraints(std::vector<MimmoSharedPointer<MimmoObject> > & extFileGeos){
574 ControlDeformExtSurface::evaluateSignedDistance(const std::vector<darray3E> &points, MimmoSharedPointer<MimmoObject> &geo,
605 skdTreeUtils::signedGlobalDistance(work.size(), work.data(), geo->getSkdTree(), suppCellIds.data(), suppCellRanks.data(), normals.data(), distanceWork.data(), sRadius, false);
607 skdTreeUtils::signedDistance(work.size(), work.data(), geo->getSkdTree(), suppCellIds.data(), distanceWork.data(), normals.data(), sRadius);
double getViolation()
Definition: ControlDeformExtSurface.cpp:126
@ SYNC
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ControlDeformExtSurface.cpp:478
double signedDistance(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *tree, long &id, std::array< double, 3 > &normal, double r)
Definition: SkdTreeUtils.cpp:84
void removeConstraintFile(std::string)
Definition: ControlDeformExtSurface.cpp:274
const fileListWithType & getConstraintFiles() const
Definition: ControlDeformExtSurface.cpp:170
ControlDeformExtSurface()
Definition: ControlDeformExtSurface.cpp:34
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
ControlDeformExtSurface is a class that check a deformation field, associated to a MimmoObject geomet...
Definition: ControlDeformExtSurface.hpp:105
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void plotOptionalResults()
Definition: ControlDeformExtSurface.cpp:652
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
std::unordered_map< std::string, int > fileListWithType
Definition: ControlDeformExtSurface.hpp:111
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
@ POINT
void addConstraintFile(std::string file, int format)
Definition: ControlDeformExtSurface.cpp:254
void setDefField(dmpvecarr3E *field)
Definition: ControlDeformExtSurface.cpp:180
virtual ~ControlDeformExtSurface()
Definition: ControlDeformExtSurface.cpp:68
void removeConstraintFiles()
Definition: ControlDeformExtSurface.cpp:282
void addConstraint(MimmoSharedPointer< MimmoObject > constraint)
Definition: ControlDeformExtSurface.cpp:220
void setConstraintFiles(fileListWithType list)
Definition: ControlDeformExtSurface.cpp:242
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
double getTolerance()
Definition: ControlDeformExtSurface.cpp:160
dmpvector1D * getViolationField()
Definition: ControlDeformExtSurface.cpp:147
ControlDeformExtSurface & operator=(ControlDeformExtSurface other)
Definition: ControlDeformExtSurface.cpp:84
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setTolerance(double tol)
Definition: ControlDeformExtSurface.cpp:209
void setGeometry(MimmoSharedPointer< MimmoObject > target)
Definition: ControlDeformExtSurface.cpp:191
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ControlDeformExtSurface.cpp:423
void swap(ControlDeformExtSurface &x) noexcept
Definition: ControlDeformExtSurface.cpp:93
void buildPorts()
Definition: ControlDeformExtSurface.cpp:106
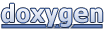