TranslationGeometry.cpp
64 TranslationGeometry::TranslationGeometry(const TranslationGeometry & other):BaseManipulation(other){
97 built = (built && createPortIn<dmpvector1D*, TranslationGeometry>(this, &mimmo::TranslationGeometry::setFilter, M_FILTER));
98 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, TranslationGeometry>(&m_geometry, M_GEOM, true));
99 built = (built && createPortOut<dmpvecarr3E*, TranslationGeometry>(this, &mimmo::TranslationGeometry::getDisplacements, M_GDISPLS));
100 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, TranslationGeometry>(this, &BaseManipulation::getGeometry, M_GEOM));
191 (*m_log)<<"Not valid filter found in "<<m_name<<". Proceeding with default unitary field"<<std::endl;
210 TranslationGeometry::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
void setFilter(dmpvector1D *filter)
Definition: TranslationGeometry.cpp:128
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: TranslationGeometry.cpp:246
void setDirection(darray3E direction)
Definition: TranslationGeometry.cpp:108
TranslationGeometry(darray3E direction={ {0, 0, 0} })
Definition: TranslationGeometry.cpp:32
TranslationGeometry & operator=(TranslationGeometry other)
Definition: TranslationGeometry.cpp:72
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: TranslationGeometry.cpp:210
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setTranslation(double alpha)
Definition: TranslationGeometry.cpp:119
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
void swap(TranslationGeometry &x) noexcept
Definition: TranslationGeometry.cpp:81
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
dmpvecarr3E * getDisplacements()
Definition: TranslationGeometry.cpp:138
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
~TranslationGeometry()
Definition: TranslationGeometry.cpp:60
TranslationGeometry is the class that applies a translation to a given geometry patch.
Definition: TranslationGeometry.hpp:75
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
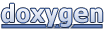