RotationGeometry.hpp
void setAxis(darray3E origin, darray3E direction)
Definition: RotationGeometry.cpp:118
void setFilter(dmpvector1D *filter)
Definition: RotationGeometry.cpp:155
RotationGeometry is the class that applies a rotation to a given geometry patch.
Definition: RotationGeometry.hpp:79
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationGeometry.cpp:255
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
RotationGeometry(darray3E origin={ {0, 0, 0} }, darray3E direction={ {0, 0, 0} })
Definition: RotationGeometry.cpp:34
dmpvecarr3E * getDisplacements()
Definition: RotationGeometry.cpp:165
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void setRotation(double alpha)
Definition: RotationGeometry.cpp:146
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationGeometry.cpp:302
void swap(RotationGeometry &x) noexcept
Definition: RotationGeometry.cpp:88
void setOrigin(darray3E origin)
Definition: RotationGeometry.cpp:127
void setDirection(darray3E direction)
Definition: RotationGeometry.cpp:135
RotationGeometry & operator=(RotationGeometry other)
Definition: RotationGeometry.cpp:79
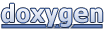