RotationGeometry.cpp
106 built = (built && createPortIn<dmpvector1D*, RotationGeometry>(this, &mimmo::RotationGeometry::setFilter, M_FILTER));
107 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, RotationGeometry>(&m_geometry, M_GEOM, true));
108 built = (built && createPortOut<dmpvecarr3E*, RotationGeometry>(this, &mimmo::RotationGeometry::getDisplacements, M_GDISPLS));
109 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, RotationGeometry>(this, &BaseManipulation::getGeometry, M_GEOM));
236 (*m_log)<<"Not valid filter found in "<<m_name<<". Proceeding with default unitary field"<<std::endl;
void setAxis(darray3E origin, darray3E direction)
Definition: RotationGeometry.cpp:118
void setFilter(dmpvector1D *filter)
Definition: RotationGeometry.cpp:155
RotationGeometry is the class that applies a rotation to a given geometry patch.
Definition: RotationGeometry.hpp:79
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationGeometry.cpp:255
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
RotationGeometry(darray3E origin={ {0, 0, 0} }, darray3E direction={ {0, 0, 0} })
Definition: RotationGeometry.cpp:34
dmpvecarr3E * getDisplacements()
Definition: RotationGeometry.cpp:165
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setRotation(double alpha)
Definition: RotationGeometry.cpp:146
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationGeometry.cpp:302
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
void swap(RotationGeometry &x) noexcept
Definition: RotationGeometry.cpp:88
@ POINT
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
void setOrigin(darray3E origin)
Definition: RotationGeometry.cpp:127
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setDirection(darray3E direction)
Definition: RotationGeometry.cpp:135
RotationGeometry & operator=(RotationGeometry other)
Definition: RotationGeometry.cpp:79
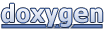