TwistGeometry.cpp
110 built = (built && createPortIn<dmpvector1D*, TwistGeometry>(this, &mimmo::TwistGeometry::setFilter, M_FILTER));
111 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, TwistGeometry>(&m_geometry, M_GEOM, true));
112 built = (built && createPortOut<dmpvecarr3E*, TwistGeometry>(this, &mimmo::TwistGeometry::getDisplacements, M_GDISPLS));
113 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, TwistGeometry>(this, &BaseManipulation::getGeometry, M_GEOM));
270 (*m_log)<<"Not valid filter found in "<<m_name<<". Proceeding with default unitary field"<<std::endl;
void setMaxDistance(double distance)
Definition: TwistGeometry.cpp:166
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: TwistGeometry.cpp:358
void setAxis(darray3E origin, darray3E direction)
Definition: TwistGeometry.cpp:122
TwistGeometry(darray3E origin={ {0, 0, 0} }, darray3E direction={ {0, 0, 0} })
Definition: TwistGeometry.cpp:32
void setDirection(darray3E direction)
Definition: TwistGeometry.cpp:139
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
dmpvecarr3E * getDisplacements()
Definition: TwistGeometry.cpp:185
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
TwistGeometry & operator=(TwistGeometry other)
Definition: TwistGeometry.cpp:81
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: TwistGeometry.cpp:289
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
TwistGeometry is the class that applies a twist to a given geometry patch.
Definition: TwistGeometry.hpp:85
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
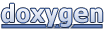