Module.cpp
94 built = (built && createPortIn<dmpvecarr3E*, Module>(this, &mimmo::Module::setField, M_VECTORFIELD, true));
95 built = (built && createPortOut<dmpvector1D*, Module>(this, &mimmo::Module::getResult, M_SCALARFIELD));
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
Module is an executable block class capable of computing the magnitude field of a vector field.
Definition: Module.hpp:66
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Module.cpp:175
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Module.cpp:163
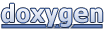