MimmoCGUtils.cpp
50 //split it in subtriangles - fixed barycenter, run 2-by-2 along vertCoords to get the other two vertices
54 distance = std::min(distance, bitpit::CGElem::distancePointTriangle(p, barycenter, vertCoords[i], vertCoords[i+1]));
67 bool isPointInsideSegment(const darray3E & p, const darray3E & V0,const darray3E & V1, double tol ){
85 bool isPointInsideTriangle(const darray3E & p, const darray3E & V0,const darray3E & V1,const darray3E & V2, double tol){
111 //split it in subtriangles - fixed vertex 0 as pivot point, run 2-by-2 along vertCoords to get the other two vertices
116 check = mimmoCGUtils::isPointInsideTriangle(p, vertCoords[0], vertCoords[i], vertCoords[i+1], tol);
double distancePointPolygon(const darray3E &p, const dvecarr3E &vertCoords)
Definition: MimmoCGUtils.cpp:41
bool isPointInsideSegment(const darray3E &p, const darray3E &V0, const darray3E &V1, double tol)
Definition: MimmoCGUtils.cpp:67
bool isPointInsidePolygon(const darray3E &p, const dvecarr3E &vertCoords, double tol)
Definition: MimmoCGUtils.cpp:109
bool isPointInsideTriangle(const darray3E &p, const darray3E &V0, const darray3E &V1, const darray3E &V2, double tol)
Definition: MimmoCGUtils.cpp:85
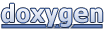