IOData.hpp
void setData(T data)
IOData is the base class of generic data stored as input or result in a Port.
Definition: IOData.hpp:39
T * getData()
IODataT is the templated class of generic data derived from IOData base class.
Definition: IOData.hpp:79
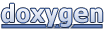