The VolCartesian defines a Cartesian patch. More...
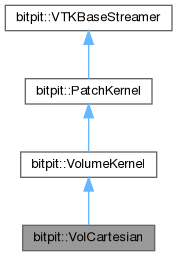

Public Types | |
enum | MemoryMode { MEMORY_NORMAL , MEMORY_LIGHT } |
![]() | |
enum | AdaptionMode { ADAPTION_DISABLED = -1 , ADAPTION_AUTOMATIC , ADAPTION_MANUAL } |
enum | AdaptionStatus { ADAPTION_CLEAN , ADAPTION_DIRTY , ADAPTION_PREPARED , ADAPTION_ALTERED } |
enum | AdjacenciesBuildStrategy { ADJACENCIES_NONE = -1 , ADJACENCIES_AUTOMATIC } |
typedef PiercedVector< Cell >::const_iterator | CellConstIterator |
typedef PiercedVector< Cell >::const_range | CellConstRange |
typedef PiercedVector< Cell >::iterator | CellIterator |
typedef PiercedVector< Cell >::range | CellRange |
typedef PiercedVector< Interface >::const_iterator | InterfaceConstIterator |
typedef PiercedVector< Interface >::const_range | InterfaceConstRange |
typedef PiercedVector< Interface >::iterator | InterfaceIterator |
typedef PiercedVector< Interface >::range | InterfaceRange |
enum | InterfacesBuildStrategy { INTERFACES_NONE = -1 , INTERFACES_AUTOMATIC } |
enum | PartitioningMode { PARTITIONING_DISABLED = -1 , PARTITIONING_ENABLED } |
enum | PartitioningStatus { PARTITIONING_CLEAN , PARTITIONING_PREPARED , PARTITIONING_ALTERED } |
typedef PiercedVector< Vertex >::const_iterator | VertexConstIterator |
typedef PiercedVector< Vertex >::const_range | VertexConstRange |
typedef PiercedVector< Vertex >::iterator | VertexIterator |
typedef PiercedVector< Vertex >::range | VertexRange |
enum | WriteTarget { WRITE_TARGET_CELLS_ALL , WRITE_TARGET_CELLS_INTERNAL } |
Public Member Functions | |
VolCartesian () | |
VolCartesian (int dimension, const std::array< double, 3 > &origin, const std::array< double, 3 > &lengths, const std::array< int, 3 > &nCells) | |
VolCartesian (int dimension, const std::array< double, 3 > &origin, double length, double dh) | |
VolCartesian (int dimension, const std::array< double, 3 > &origin, double length, int nCells1D) | |
VolCartesian (int id, int dimension, const std::array< double, 3 > &origin, const std::array< double, 3 > &lengths, const std::array< int, 3 > &nCells) | |
VolCartesian (int id, int dimension, const std::array< double, 3 > &origin, double length, double dh) | |
VolCartesian (int id, int dimension, const std::array< double, 3 > &origin, double length, int nCells1D) | |
VolCartesian (std::istream &stream) | |
std::unique_ptr< PatchKernel > | clone () const override |
std::vector< double > | convertToCellData (const std::vector< double > &vertexData) const |
std::vector< double > | convertToVertexData (const std::vector< double > &cellData) const |
void | evalCellBoundingBox (long id, std::array< double, 3 > *minPoint, std::array< double, 3 > *maxPoint) const override |
std::array< double, 3 > | evalCellCentroid (const std::array< int, 3 > &ijk) const |
std::array< double, 3 > | evalCellCentroid (long id) const override |
double | evalCellSize (const std::array< int, 3 > &ijk) const |
double | evalCellSize (long id) const override |
double | evalCellVolume (const std::array< int, 3 > &ijk) const |
double | evalCellVolume (long id) const override |
double | evalInterfaceArea (long id) const override |
std::array< double, 3 > | evalInterfaceNormal (long id) const override |
std::array< double, 3 > | evalVertexCoords (const std::array< int, 3 > &ijk) const |
std::array< double, 3 > | evalVertexCoords (long id) const |
std::vector< long > | extractCellSubSet (int idMin, int idMax) const |
std::vector< long > | extractCellSubSet (std::array< double, 3 > const &pointMin, std::array< double, 3 > const &pointMax) const |
std::vector< long > | extractCellSubSet (std::array< int, 3 > const &ijkMin, std::array< int, 3 > const &ijkMax) const |
std::vector< long > | extractVertexSubSet (int idMin, int idMax) const |
std::vector< long > | extractVertexSubSet (std::array< double, 3 > const &pointMin, std::array< double, 3 > const &pointMax) const |
std::vector< long > | extractVertexSubSet (std::array< int, 3 > const &ijkMin, std::array< int, 3 > const &ijkMax) const |
std::array< int, 3 > | getCellCartesianId (long id) const |
const std::vector< double > & | getCellCentroids (int direction) const |
long | getCellCount () const override |
int | getCellCount (int direction) const |
std::array< int, 3 > | getCellFaceNeighsCartesianId (long id, int face) const |
long | getCellFaceNeighsLinearId (long id, int face) const |
long | getCellLinearId (const std::array< int, 3 > &ijk) const |
long | getCellLinearId (int i, int j, int k) const |
ElementType | getCellType () const |
ElementType | getCellType (long id) const override |
long | getInterfaceCount () const override |
ElementType | getInterfaceType () const |
ElementType | getInterfaceType (long id) const override |
std::array< double, 3 > | getLengths () const |
MemoryMode | getMemoryMode () const |
std::array< double, 3 > | getOrigin () const |
std::array< double, 3 > | getSpacing () const |
double | getSpacing (int direction) const |
std::array< int, 3 > | getVertexCartesianId (const std::array< int, 3 > &cellIjk, int vertex) const |
std::array< int, 3 > | getVertexCartesianId (long cellId, int vertex) const |
std::array< int, 3 > | getVertexCartesianId (long id) const |
const std::vector< double > & | getVertexCoords (int direction) const |
long | getVertexCount () const override |
int | getVertexCount (int direction) const |
long | getVertexLinearId (const std::array< int, 3 > &ijk) const |
long | getVertexLinearId (int i, int j, int k) const |
bool | isCellCartesianIdValid (const std::array< int, 3 > &ijk) const |
bool | isPointInside (const std::array< double, 3 > &point) const override |
bool | isPointInside (double x, double y, double z) const |
bool | isPointInside (long id, const std::array< double, 3 > &point) const override |
bool | isPointInside (long id, double x, double y, double z) const |
bool | isVertexCartesianIdValid (const std::array< int, 3 > &ijk) const |
int | linearCellInterpolation (const std::array< double, 3 > &point, std::vector< int > *stencil, std::vector< double > *weights) const |
int | linearVertexInterpolation (const std::array< double, 3 > &point, std::vector< int > *stencil, std::vector< double > *weights) const |
long | locateClosestCell (std::array< double, 3 > const &point) const |
std::array< int, 3 > | locateClosestCellCartesian (std::array< double, 3 > const &point) const |
long | locateClosestVertex (std::array< double, 3 > const &point) const |
std::array< int, 3 > | locateClosestVertexCartesian (std::array< double, 3 > const &point) const |
long | locatePoint (const std::array< double, 3 > &point) const override |
long | locatePoint (double x, double y, double z) const |
std::array< int, 3 > | locatePointCartesian (const std::array< double, 3 > &point) const |
void | reset () override |
void | rotate (const std::array< double, 3 > &n0, const std::array< double, 3 > &n1, double angle) override |
void | scale (const std::array< double, 3 > &scaling, const std::array< double, 3 > ¢er) override |
void | setLengths (const std::array< double, 3 > &lengths) |
void | setOrigin (const std::array< double, 3 > &origin) |
void | switchMemoryMode (MemoryMode mode) |
void | translate (const std::array< double, 3 > &translation) override |
![]() | |
bool | areFaceVerticesOrdered (const Cell &cell, int face) const |
void | extractEnvelope (SurfaceKernel &envelope) const |
int | getFaceOrderedLocalVertex (const Cell &cell, int face, std::size_t n) const |
ConstProxyVector< long > | getFaceOrderedVertexIds (const Cell &cell, int face) const |
int | getLineCodimension () const override |
int | getPointCodimension () const override |
int | getSurfaceCodimension () const override |
int | getVolumeCodimension () const override |
bool | isPointInside (double x, double y, double z) const |
bool | isPointInside (long id, double x, double y, double z) const |
![]() | |
PatchKernel (PatchKernel &&other) | |
virtual | ~PatchKernel () |
std::vector< adaption::Info > | adaption (bool trackAdaption=true, bool squeezeStorage=false) |
std::vector< adaption::Info > | adaptionAlter (bool trackAdaption=true, bool squeezeStorage=false) |
void | adaptionCleanup () |
std::vector< adaption::Info > | adaptionPrepare (bool trackAdaption=true) |
CellIterator | addCell (Cell &&source, int owner, int haloLayer, long id=Element::NULL_ID) |
CellIterator | addCell (Cell &&source, int owner, long id=Element::NULL_ID) |
CellIterator | addCell (Cell &&source, long id=Element::NULL_ID) |
CellIterator | addCell (const Cell &source, int owner, int haloLayer, long id=Element::NULL_ID) |
CellIterator | addCell (const Cell &source, int owner, long id=Element::NULL_ID) |
CellIterator | addCell (const Cell &source, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, const std::vector< long > &connectivity, int owner, int haloLayer, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, const std::vector< long > &connectivity, int owner, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, const std::vector< long > &connectivity, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, int owner, int haloLayer, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, int owner, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, std::unique_ptr< long[]> &&connectStorage, int owner, int haloLayer, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, std::unique_ptr< long[]> &&connectStorage, int owner, long id=Element::NULL_ID) |
CellIterator | addCell (ElementType type, std::unique_ptr< long[]> &&connectStorage, long id=Element::NULL_ID) |
InterfaceIterator | addInterface (const Interface &source, long id=Element::NULL_ID) |
InterfaceIterator | addInterface (ElementType type, const std::vector< long > &connectivity, long id=Element::NULL_ID) |
InterfaceIterator | addInterface (ElementType type, long id=Element::NULL_ID) |
InterfaceIterator | addInterface (ElementType type, std::unique_ptr< long[]> &&connectStorage, long id=Element::NULL_ID) |
InterfaceIterator | addInterface (Interface &&source, long id=Element::NULL_ID) |
VertexIterator | addVertex (const std::array< double, 3 > &coords, long id=Vertex::NULL_ID) |
VertexIterator | addVertex (const Vertex &source, long id=Vertex::NULL_ID) |
VertexIterator | addVertex (Vertex &&source, long id=Vertex::NULL_ID) |
bool | areAdjacenciesDirty (bool global=false) const |
bool | areInterfacesDirty (bool global=false) const |
bool | arePartitioningInfoDirty (bool global=true) const |
void | buildAdjacencies () |
void | buildInterfaces () |
CellIterator | cellBegin () |
CellConstIterator | cellConstBegin () const |
CellConstIterator | cellConstEnd () const |
CellIterator | cellEnd () |
std::vector< long > | collapseCoincidentVertices () |
void | consecutiveRenumber (long offsetVertices, long offsetCells, long offsetInterfaces) |
void | consecutiveRenumberCells (long offset=0) |
void | consecutiveRenumberInterfaces (long offset=0) |
void | consecutiveRenumberVertices (long offset=0) |
long | countBorderCells () const |
long | countBorderFaces () const |
long | countBorderInterfaces () const |
long | countBorderVertices () const |
long | countDuplicateCells () const |
long | countFaces () const |
long | countFreeCells () const |
long | countFreeFaces () const |
long | countFreeInterfaces () const |
long | countFreeVertices () const |
long | countOrphanCells () const |
long | countOrphanInterfaces () const |
long | countOrphanVertices () const |
bool | deleteCell (long id) |
template<typename IdStorage> | |
bool | deleteCells (const IdStorage &ids) |
bool | deleteCoincidentVertices () |
bool | deleteInterface (long id) |
template<typename IdStorage> | |
bool | deleteInterfaces (const IdStorage &ids) |
bool | deleteOrphanInterfaces () |
bool | deleteOrphanVertices () |
void | destroyAdjacencies () |
void | destroyInterfaces () |
void | displayCells (std::ostream &out, unsigned int padding=0) const |
void | displayInterfaces (std::ostream &out, unsigned int padding=0) const |
void | displayTopologyStats (std::ostream &out, unsigned int padding=0) const |
void | displayVertices (std::ostream &out, unsigned int padding=0) const |
bool | dump (std::ostream &stream) |
bool | dump (std::ostream &stream) const |
bool | empty (bool global=true) const |
void | enableCellBalancing (long id, bool enabled) |
void | evalElementBoundingBox (const Element &element, std::array< double, 3 > *minPoint, std::array< double, 3 > *maxPoint) const |
std::array< double, 3 > | evalElementCentroid (const Element &element) const |
virtual void | evalInterfaceBoundingBox (long id, std::array< double, 3 > *minPoint, std::array< double, 3 > *maxPoint) const |
virtual std::array< double, 3 > | evalInterfaceCentroid (long id) const |
double | evalPartitioningUnbalance () const |
double | evalPartitioningUnbalance (const std::unordered_map< long, double > &cellWeights) const |
void | findCellEdgeNeighs (long id, bool complete, std::vector< long > *neighs) const |
std::vector< long > | findCellEdgeNeighs (long id, bool complete=true) const |
std::vector< long > | findCellEdgeNeighs (long id, int edge) const |
void | findCellEdgeNeighs (long id, int edge, std::vector< long > *neighs) const |
std::vector< long > | findCellFaceNeighs (long id) const |
std::vector< long > | findCellFaceNeighs (long id, int face) const |
void | findCellFaceNeighs (long id, int face, std::vector< long > *neighs) const |
void | findCellFaceNeighs (long id, std::vector< long > *neighs) const |
std::vector< long > | findCellNeighs (long id) const |
void | findCellNeighs (long id, int codimension, bool complete, std::vector< long > *neighs) const |
std::vector< long > | findCellNeighs (long id, int codimension, bool complete=true) const |
void | findCellNeighs (long id, std::vector< long > *neighs) const |
void | findCellVertexNeighs (long id, bool complete, std::vector< long > *neighs) const |
std::vector< long > | findCellVertexNeighs (long id, bool complete=true) const |
std::vector< long > | findCellVertexNeighs (long id, int vertex) const |
void | findCellVertexNeighs (long id, int vertex, std::vector< long > *neighs) const |
std::vector< long > | findCellVertexOneRing (long id, int vertex) const |
void | findCellVertexOneRing (long id, int vertex, std::vector< long > *neighs) const |
std::vector< long > | findDuplicateCells () const |
bool | findFaceNeighCell (long cellId, long neighId, int *cellFace, int *cellAdjacencyId) const |
std::vector< long > | findOrphanCells () const |
std::vector< long > | findOrphanInterfaces () const |
std::vector< long > | findOrphanVertices () |
std::vector< long > | findVertexOneRing (long vertexId) const |
void | findVertexOneRing (long vertexId, std::vector< long > *ring) const |
void | flushData (std::fstream &stream, const std::string &name, VTKFormat format) override |
AdaptionMode | getAdaptionMode () const |
AdaptionStatus | getAdaptionStatus (bool global=false) const |
AdjacenciesBuildStrategy | getAdjacenciesBuildStrategy () const |
void | getBoundingBox (bool global, std::array< double, 3 > &minPoint, std::array< double, 3 > &maxPoint) const |
void | getBoundingBox (std::array< double, 3 > &minPoint, std::array< double, 3 > &maxPoint) const |
Cell & | getCell (long id) |
const Cell & | getCell (long id) const |
adaption::Marker | getCellAdaptionMarker (long id) |
CellConstIterator | getCellConstIterator (long id) const |
int | getCellHaloLayer (long id) const |
CellIterator | getCellIterator (long id) |
int | getCellOwner (long id) const |
int | getCellRank (long id) const |
PiercedVector< Cell > & | getCells () |
const PiercedVector< Cell > & | getCells () const |
ConstProxyVector< std::array< double BITPIT_COMMA 3 > > | getCellVertexCoordinates (long id) const |
void | getCellVertexCoordinates (long id, std::array< double, 3 > *coordinates) const |
void | getCellVertexCoordinates (long id, std::unique_ptr< std::array< double, 3 >[]> *coordinates) const |
const MPI_Comm & | getCommunicator () const |
int | getDimension () const |
int | getDumpVersion () const |
ConstProxyVector< std::array< double BITPIT_COMMA 3 > > | getElementVertexCoordinates (const Element &element) const |
void | getElementVertexCoordinates (const Element &element, std::array< double, 3 > *coordinates) const |
void | getElementVertexCoordinates (const Element &element, std::unique_ptr< std::array< double, 3 >[]> *coordinates) const |
Cell & | getFirstGhost () |
const Cell & | getFirstGhost () const |
Cell & | getFirstGhostCell () |
const Cell & | getFirstGhostCell () const |
Vertex & | getFirstGhostVertex () |
const Vertex & | getFirstGhostVertex () const |
long | getGhostCellCount () const |
const std::unordered_map< int, std::vector< long > > & | getGhostCellExchangeSources () const |
const std::vector< long > & | getGhostCellExchangeSources (int rank) const |
const std::unordered_map< int, std::vector< long > > & | getGhostCellExchangeTargets () const |
const std::vector< long > & | getGhostCellExchangeTargets (int rank) const |
long | getGhostCount () const |
const std::unordered_map< int BITPIT_COMMA std::vector< long > > & | getGhostExchangeSources () const |
const std::vector< long > & | getGhostExchangeSources (int rank) const |
const std::unordered_map< int BITPIT_COMMA std::vector< long > > & | getGhostExchangeTargets () const |
const std::vector< long > & | getGhostExchangeTargets (int rank) const |
long | getGhostVertexCount () const |
const std::unordered_map< int, std::vector< long > > & | getGhostVertexExchangeSources () const |
const std::vector< long > & | getGhostVertexExchangeSources (int rank) const |
const std::unordered_map< int, std::vector< long > > & | getGhostVertexExchangeTargets () const |
const std::vector< long > & | getGhostVertexExchangeTargets (int rank) const |
std::size_t | getHaloSize () const |
int | getId () const |
Interface & | getInterface (long id) |
const Interface & | getInterface (long id) const |
InterfaceConstIterator | getInterfaceConstIterator (long id) const |
InterfaceIterator | getInterfaceIterator (long id) |
PiercedVector< Interface > & | getInterfaces () |
const PiercedVector< Interface > & | getInterfaces () const |
InterfacesBuildStrategy | getInterfacesBuildStrategy () const |
ConstProxyVector< std::array< double BITPIT_COMMA 3 > > | getInterfaceVertexCoordinates (long id) const |
void | getInterfaceVertexCoordinates (long id, std::array< double, 3 > *coordinates) const |
void | getInterfaceVertexCoordinates (long id, std::unique_ptr< std::array< double, 3 >[]> *coordinates) const |
long | getInternalCellCount () const |
std::set< int > | getInternalCellPIDs () |
std::vector< long > | getInternalCellsByPID (int pid) |
long | getInternalCount () const |
long | getInternalVertexCount () const |
Cell & | getLastInternalCell () |
const Cell & | getLastInternalCell () const |
Vertex & | getLastInternalVertex () |
const Vertex & | getLastInternalVertex () const |
std::vector< int > | getNeighbourRanks () |
int | getOwner (bool allowDirty=false) const |
PartitioningMode | getPartitioningMode () const |
PartitioningStatus | getPartitioningStatus (bool global=false) const |
int | getProcessorCount () const |
int | getRank () const |
double | getTol () const |
Vertex & | getVertex (long id) |
const Vertex & | getVertex (long id) const |
VertexConstIterator | getVertexConstIterator (long id) const |
const std::array< double, 3 > & | getVertexCoords (long id) const |
void | getVertexCoords (std::size_t nVertices, const long *ids, std::array< double, 3 > *coordinates) const |
void | getVertexCoords (std::size_t nVertices, const long *ids, std::unique_ptr< std::array< double, 3 >[]> *coordinates) const |
VertexIterator | getVertexIterator (long id) |
int | getVertexOwner (long id) const |
int | getVertexRank (long id) const |
PiercedVector< Vertex > & | getVertices () |
const PiercedVector< Vertex > & | getVertices () const |
VTKUnstructuredGrid & | getVTK () |
const VTKUnstructuredGrid & | getVTK () const |
const CellConstRange | getVTKCellWriteRange () const |
WriteTarget | getVTKWriteTarget () const |
CellIterator | ghostBegin () |
CellIterator | ghostCell2InternalCell (long id) |
CellIterator | ghostCellBegin () |
CellConstIterator | ghostCellConstBegin () const |
CellConstIterator | ghostCellConstEnd () const |
CellIterator | ghostCellEnd () |
CellConstIterator | ghostConstBegin () const |
CellConstIterator | ghostConstEnd () const |
CellIterator | ghostEnd () |
VertexIterator | ghostVertexBegin () |
VertexConstIterator | ghostVertexConstBegin () const |
VertexConstIterator | ghostVertexConstEnd () const |
VertexIterator | ghostVertexEnd () |
void | initializeAdjacencies (AdjacenciesBuildStrategy strategy=ADJACENCIES_AUTOMATIC) |
void | initializeInterfaces (InterfacesBuildStrategy strategy=INTERFACES_AUTOMATIC) |
InterfaceIterator | interfaceBegin () |
InterfaceConstIterator | interfaceConstBegin () const |
InterfaceConstIterator | interfaceConstEnd () const |
InterfaceIterator | interfaceEnd () |
CellIterator | internalBegin () |
CellIterator | internalCell2GhostCell (long id, int owner, int haloLayer) |
CellIterator | internalCellBegin () |
CellConstIterator | internalCellConstBegin () const |
CellConstIterator | internalCellConstEnd () const |
CellIterator | internalCellEnd () |
CellConstIterator | internalConstBegin () const |
CellConstIterator | internalConstEnd () const |
CellIterator | internalEnd () |
VertexIterator | internalVertexBegin () |
VertexConstIterator | internalVertexConstBegin () const |
VertexConstIterator | internalVertexConstEnd () const |
VertexIterator | internalVertexEnd () |
bool | intersectInterfacePlane (long id, std::array< double, 3 > P, std::array< double, 3 > nP, std::array< std::array< double, 3 >, 2 > *intersection, std::vector< std::array< double, 3 > > *polygon) const |
bool | isAdaptionSupported () const |
bool | isBoundingBoxDirty (bool global=false) const |
bool | isCellAutoIndexingEnabled () const |
bool | isDirty (bool global=false) const |
bool | isDistributed (bool allowDirty=false) const |
bool | isExpert () const |
bool | isInterfaceAutoIndexingEnabled () const |
bool | isInterfaceOrphan (long id) const |
bool | isPartitioned () const |
bool | isPartitioningSupported () const |
bool | isRankNeighbour (int rank) |
bool | isThreeDimensional () const |
bool | isTolCustomized () const |
bool | isVertexAutoIndexingEnabled () const |
long | locatePoint (double x, double y, double z) const |
void | markCellForCoarsening (long id) |
void | markCellForRefinement (long id) |
PatchKernel & | operator= (PatchKernel &&other) |
std::vector< adaption::Info > | partition (bool trackPartitioning, bool squeezeStorage=false) |
std::vector< adaption::Info > | partition (const std::unordered_map< long, double > &cellWeights, bool trackPartitioning, bool squeezeStorage=false) |
std::vector< adaption::Info > | partition (const std::unordered_map< long, int > &cellRanks, bool trackPartitioning, bool squeezeStorage=false) |
std::vector< adaption::Info > | partition (MPI_Comm communicator, bool trackPartitioning, bool squeezeStorage=false, std::size_t haloSize=1) |
std::vector< adaption::Info > | partition (MPI_Comm communicator, const std::unordered_map< long, double > &cellWeights, bool trackPartitioning, bool squeezeStorage=false, std::size_t haloSize=1) |
std::vector< adaption::Info > | partition (MPI_Comm communicator, const std::unordered_map< long, int > &cellRanks, bool trackPartitioning, bool squeezeStorage=false, std::size_t haloSize=1) |
std::vector< adaption::Info > | partitioningAlter (bool trackPartitioning=true, bool squeezeStorage=false) |
void | partitioningCleanup () |
std::vector< adaption::Info > | partitioningPrepare (bool trackPartitioning) |
std::vector< adaption::Info > | partitioningPrepare (const std::unordered_map< long, double > &cellWeights, bool trackPartitioning) |
std::vector< adaption::Info > | partitioningPrepare (const std::unordered_map< long, int > &cellRanks, bool trackPartitioning) |
std::vector< adaption::Info > | partitioningPrepare (MPI_Comm communicator, bool trackPartitioning, std::size_t haloSize=1) |
std::vector< adaption::Info > | partitioningPrepare (MPI_Comm communicator, const std::unordered_map< long, double > &cellWeights, bool trackPartitioning, std::size_t haloSize=1) |
std::vector< adaption::Info > | partitioningPrepare (MPI_Comm communicator, const std::unordered_map< long, int > &cellRanks, bool trackPartitioning, std::size_t haloSize=1) |
template<typename Function> | |
void | processCellFaceNeighbours (long seedId, int nLayers, Function function) const |
template<typename Selector, typename Function> | |
void | processCellFaceNeighbours (long seedId, int nLayers, Selector isSelected, Function function) const |
template<typename Function> | |
void | processCellNeighbours (long seedId, int nLayers, Function function) const |
template<typename Selector, typename Function> | |
void | processCellNeighbours (long seedId, int nLayers, Selector isSelected, Function function) const |
template<typename Function, typename SeedContainer> | |
void | processCellsFaceNeighbours (const SeedContainer &seedIds, int nLayers, Function function) const |
template<typename Selector, typename Function, typename SeedContainer> | |
void | processCellsFaceNeighbours (const SeedContainer &seedIds, int nLayers, Selector isSelected, Function function) const |
template<typename Function, typename SeedContainer> | |
void | processCellsNeighbours (const SeedContainer &seedIds, int nLayers, Function function) const |
template<typename Selector, typename Function, typename SeedContainer> | |
void | processCellsNeighbours (const SeedContainer &seedIds, int nLayers, Selector isSelected, Function function) const |
bool | reserveCells (size_t nCells) |
bool | reserveInterfaces (size_t nInterfaces) |
bool | reserveVertices (size_t nVertices) |
void | resetCellAdaptionMarker (long id) |
virtual void | resetCells () |
virtual void | resetInterfaces () |
void | resetTol () |
virtual void | resetVertices () |
void | restore (std::istream &stream, bool reregister=false) |
void | rotate (double n0x, double n0y, double n0z, double n1x, double n1y, double n1z, double angle) |
void | scale (const std::array< double, 3 > &scaling) |
void | scale (double scaling) |
void | scale (double scaling, const std::array< double, 3 > &origin) |
void | scale (double sx, double sy, double sz) |
void | scale (double sx, double sy, double sz, const std::array< double, 3 > &origin) |
void | setCellAutoIndexing (bool enabled) |
virtual void | setDimension (int dimension) |
void | setHaloSize (std::size_t haloSize) |
void | setId (int id) |
void | setInterfaceAutoIndexing (bool enabled) |
virtual void | settleAdaptionMarkers () |
void | setTol (double tolerance) |
void | setVertexAutoIndexing (bool enabled) |
void | setVTKWriteTarget (WriteTarget targetCells) |
virtual void | simulateCellUpdate (const long id, adaption::Marker marker, std::vector< Cell > *virtualCells, PiercedVector< Vertex, long > *virtualVertices) const |
bool | sort () |
bool | sortCells () |
bool | sortInterfaces () |
bool | sortVertices () |
bool | squeeze () |
bool | squeezeCells () |
bool | squeezeInterfaces () |
bool | squeezeVertices () |
void | translate (double sx, double sy, double sz) |
std::vector< adaption::Info > | update (bool trackAdaption=true, bool squeezeStorage=false) |
void | updateAdjacencies (bool forcedUpdated=false) |
void | updateBoundingBox (bool forcedUpdated=false) |
void | updateInterfaces (bool forcedUpdated=false) |
VertexIterator | vertexBegin () |
VertexConstIterator | vertexConstBegin () const |
VertexConstIterator | vertexConstEnd () const |
VertexIterator | vertexEnd () |
void | write (const std::string &name, VTKWriteMode mode, double time) |
void | write (const std::string &name, VTKWriteMode mode=VTKWriteMode::DEFAULT) |
void | write (VTKWriteMode mode, double time) |
void | write (VTKWriteMode mode=VTKWriteMode::DEFAULT) |
![]() | |
virtual void | absorbData (std::fstream &, const std::string &, VTKFormat, uint64_t, uint8_t, VTKDataType) |
template<typename T> | |
void | flushValue (std::fstream &, VTKFormat, const T &value) const |
template<typename T> | |
void | flushValue (std::fstream &, VTKFormat, const T *values, int nValues) const |
Protected Member Functions | |
void | _dump (std::ostream &stream) const override |
void | _findCellEdgeNeighs (long id, int edge, const std::vector< long > *blackList, std::vector< long > *neighs) const override |
void | _findCellFaceNeighs (long id, int face, const std::vector< long > *blackList, std::vector< long > *neighs) const override |
void | _findCellVertexNeighs (long id, int vertex, const std::vector< long > *blackList, std::vector< long > *neighs) const override |
int | _getDumpVersion () const override |
void | _restore (std::istream &stream) override |
void | _updateAdjacencies () override |
void | _updateInterfaces () override |
![]() | |
VolumeKernel (int dimension, MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
VolumeKernel (int id, int dimension, MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
VolumeKernel (MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
![]() | |
PatchKernel (const PatchKernel &other) | |
PatchKernel (int dimension, MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
PatchKernel (int id, int dimension, MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
PatchKernel (MPI_Comm communicator, std::size_t haloSize, AdaptionMode adaptionMode, PartitioningMode partitioningMode) | |
virtual std::vector< adaption::Info > | _adaptionAlter (bool trackAdaption) |
virtual void | _adaptionCleanup () |
virtual std::vector< adaption::Info > | _adaptionPrepare (bool trackAdaption) |
virtual bool | _enableCellBalancing (long id, bool enabled) |
virtual void | _findCellNeighs (long id, const std::vector< long > *blackList, std::vector< long > *neighs) const |
virtual std::vector< long > | _findGhostCellExchangeSources (int rank) |
virtual adaption::Marker | _getCellAdaptionMarker (long id) |
virtual long | _getCellNativeIndex (long id) const |
virtual std::size_t | _getMaxHaloSize () |
virtual bool | _markCellForCoarsening (long id) |
virtual bool | _markCellForRefinement (long id) |
virtual std::vector< adaption::Info > | _partitioningAlter (bool trackPartitioning) |
virtual void | _partitioningCleanup () |
virtual std::vector< adaption::Info > | _partitioningPrepare (const std::unordered_map< long, double > &cellWeights, double defaultWeight, bool trackPartitioning) |
virtual std::vector< adaption::Info > | _partitioningPrepare (const std::unordered_map< long, int > &cellRanks, bool trackPartitioning) |
virtual void | _resetAdjacencies (bool release) |
virtual bool | _resetCellAdaptionMarker (long id) |
virtual void | _resetInterfaces (bool release) |
virtual void | _resetTol () |
virtual void | _setHaloSize (std::size_t haloSize) |
virtual void | _setTol (double tolerance) |
virtual void | _writeFinalize () |
virtual void | _writePrepare () |
void | addPointToBoundingBox (const std::array< double, 3 > &point) |
std::unordered_map< long, std::vector< long > > | binGroupVertices (const PiercedVector< Vertex > &vertices, int nBins) |
std::unordered_map< long, std::vector< long > > | binGroupVertices (int nBins) |
void | clearBoundingBox () |
template<typename item_t, typename id_t = long> | |
std::unordered_map< id_t, id_t > | consecutiveItemRenumbering (PiercedVector< item_t, id_t > &container, long offset) |
bool | deleteVertex (long id) |
template<typename IdStorage> | |
bool | deleteVertices (const IdStorage &ids) |
void | dumpCells (std::ostream &stream) const |
void | dumpInterfaces (std::ostream &stream) const |
void | dumpVertices (std::ostream &stream) const |
void | extractEnvelope (PatchKernel &envelope) const |
virtual int | findAdjoinNeighFace (const Cell &cell, int cellFace, const Cell &neigh) const |
AlterationFlags | getCellAlterationFlags (long id) const |
AlterationFlags | getInterfaceAlterationFlags (long id) const |
VertexIterator | ghostVertex2InternalVertex (long id) |
VertexIterator | internalVertex2GhostVertex (long id, int owner) |
bool | isBoundingBoxFrozen () const |
bool | isCommunicatorSet () const |
virtual bool | isSameFace (const Cell &cell_A, int face_A, const Cell &cell_B, int face_B) const |
template<typename item_t, typename id_t = long> | |
void | mappedItemRenumbering (PiercedVector< item_t, id_t > &container, const std::unordered_map< id_t, id_t > &renumberMap) |
PatchKernel & | operator= (const PatchKernel &other)=delete |
void | pruneStaleAdjacencies () |
void | pruneStaleInterfaces () |
void | removePointFromBoundingBox (const std::array< double, 3 > &point) |
void | resetAdjacencies () |
void | resetCellAlterationFlags (long id, AlterationFlags flags=FLAG_NONE) |
void | resetInterfaceAlterationFlags (long id, AlterationFlags flags=FLAG_NONE) |
CellIterator | restoreCell (ElementType type, std::unique_ptr< long[]> &&connectStorage, int owner, int haloLayer, long id) |
void | restoreCells (std::istream &stream) |
InterfaceIterator | restoreInterface (ElementType type, std::unique_ptr< long[]> &&connectStorage, long id) |
void | restoreInterfaces (std::istream &stream) |
VertexIterator | restoreVertex (const std::array< double, 3 > &coords, int owner, long id) |
void | restoreVertices (std::istream &stream) |
void | setAdaptionMode (AdaptionMode mode) |
void | setAdaptionStatus (AdaptionStatus status) |
void | setAdjacenciesBuildStrategy (AdjacenciesBuildStrategy status) |
void | setBoundingBox (const std::array< double, 3 > &minPoint, const std::array< double, 3 > &maxPoint) |
void | setBoundingBoxDirty (bool dirty) |
void | setBoundingBoxFrozen (bool frozen) |
void | setCellAlterationFlags (AlterationFlags flags) |
void | setCellAlterationFlags (long id, AlterationFlags flags) |
virtual void | setCommunicator (MPI_Comm communicator) |
void | setExpert (bool expert) |
void | setInterfaceAlterationFlags (AlterationFlags flags) |
void | setInterfaceAlterationFlags (long id, AlterationFlags flags) |
void | setInterfacesBuildStrategy (InterfacesBuildStrategy status) |
void | setPartitioned (bool partitioned) |
void | setPartitioningMode (PartitioningMode mode) |
void | setPartitioningStatus (PartitioningStatus status) |
bool | testAlterationFlags (AlterationFlags availableFlags, AlterationFlags requestedFlags) const |
bool | testCellAlterationFlags (long id, AlterationFlags flags) const |
bool | testInterfaceAlterationFlags (long id, AlterationFlags flags) const |
void | unsetCellAlterationFlags (AlterationFlags flags) |
void | unsetCellAlterationFlags (long id, AlterationFlags flags) |
void | unsetInterfaceAlterationFlags (AlterationFlags flags) |
void | unsetInterfaceAlterationFlags (long id, AlterationFlags flags) |
void | updateFirstGhostCellId () |
void | updateFirstGhostVertexId () |
void | updateLastInternalCellId () |
void | updateLastInternalVertexId () |
Additional Inherited Members | |
![]() | |
template<typename patch_t> | |
static std::unique_ptr< patch_t > | clone (const patch_t *original) |
![]() | |
typedef uint16_t | AlterationFlags |
typedef std::unordered_map< long, AlterationFlags > | AlterationFlagsStorage |
![]() | |
AlterationFlagsStorage | m_alteredCells |
AlterationFlagsStorage | m_alteredInterfaces |
PiercedVector< Cell > | m_cells |
PiercedVector< Interface > | m_interfaces |
PiercedVector< Vertex > | m_vertices |
![]() | |
static const double | DEFAULT_PARTITIONING_WEIGTH = 1. |
static const AlterationFlags | FLAG_ADJACENCIES_DIRTY = (1u << 1) |
static const AlterationFlags | FLAG_DANGLING = (1u << 3) |
static const AlterationFlags | FLAG_DELETED = (1u << 0) |
static const AlterationFlags | FLAG_INTERFACES_DIRTY = (1u << 2) |
static const AlterationFlags | FLAG_NONE = 0x0 |
Detailed Description
The VolCartesian defines a Cartesian patch.
VolCartesian is a Cartesian patch that can operate in two modes. In normal memory mode, the patch behaves like a standard patch: all data structures needed by the patch are generated and stored within the ptch. This means that, in normal mode, the patch will create cells, vertices and, if requested, interfaces data structures. In light memory mode, the patch will create only a minimal set of data structures. Altohugh this allows to reduce its memory footprint, it will also limit the functionalites available when operating in light memory mode. Features that are available in light memory mode are:
- evaluation of vertex/cell/interface topological properties;
- evaluation of vertex/cell/interface geometrical properties;
- evaluation of cell neighbours. Features that are NOT available in light memory mode are:
- access to vertex/cell/interface data structures;
- access to vertex/cell/interface iterators;
- writing of VTK files. When in light memory mode, updating the patch will automatically switch to normal memory mode. A newly constructed patch will operate in light memory mode.
- Examples
- volcartesian_example_00001.cpp.
Definition at line 37 of file volcartesian.hpp.
Member Enumeration Documentation
◆ MemoryMode
enum bitpit::VolCartesian::MemoryMode |
Definition at line 45 of file volcartesian.hpp.
Constructor & Destructor Documentation
◆ VolCartesian() [1/8]
bitpit::VolCartesian::VolCartesian | ( | ) |
Creates an uninitialized patch.
Definition at line 67 of file volcartesian.cpp.
◆ VolCartesian() [2/8]
bitpit::VolCartesian::VolCartesian | ( | int | dimension, |
const std::array< double, 3 > & | origin, | ||
const std::array< double, 3 > & | lengths, | ||
const std::array< int, 3 > & | nCells ) |
Creates a patch.
- Parameters
-
dimension is the dimension of the patch origin is the origin of the domain lengths are the lengths of the domain nCells are the numbers of cells of the patch
Definition at line 85 of file volcartesian.cpp.
◆ VolCartesian() [3/8]
bitpit::VolCartesian::VolCartesian | ( | int | id, |
int | dimension, | ||
const std::array< double, 3 > & | origin, | ||
const std::array< double, 3 > & | lengths, | ||
const std::array< int, 3 > & | nCells ) |
Creates a patch.
- Parameters
-
id is the id of the patch dimension is the dimension of the patch origin is the origin of the domain lengths are the lengths of the domain nCells are the number of cells along each direction
Definition at line 102 of file volcartesian.cpp.
◆ VolCartesian() [4/8]
bitpit::VolCartesian::VolCartesian | ( | int | dimension, |
const std::array< double, 3 > & | origin, | ||
double | length, | ||
int | nCells ) |
Creates a patch.
- Parameters
-
dimension is the dimension of the patch origin is the origin of the domain length is the length of the domain nCells is the number of cells along each direction
Definition at line 128 of file volcartesian.cpp.
◆ VolCartesian() [5/8]
bitpit::VolCartesian::VolCartesian | ( | int | id, |
int | dimension, | ||
const std::array< double, 3 > & | origin, | ||
double | length, | ||
int | nCells ) |
Creates a patch.
- Parameters
-
id is the id of the patch dimension is the dimension of the patch origin is the origin of the domain length is the length of the domain nCells is the number of cells along each direction
Definition at line 144 of file volcartesian.cpp.
◆ VolCartesian() [6/8]
bitpit::VolCartesian::VolCartesian | ( | int | dimension, |
const std::array< double, 3 > & | origin, | ||
double | length, | ||
double | dh ) |
Creates a patch.
- Parameters
-
dimension is the dimension of the patch origin is the origin of the domain length is the length of the domain dh is the maximum allowed mesh spacing
Definition at line 169 of file volcartesian.cpp.
◆ VolCartesian() [7/8]
bitpit::VolCartesian::VolCartesian | ( | int | id, |
int | dimension, | ||
const std::array< double, 3 > & | origin, | ||
double | length, | ||
double | dh ) |
Creates a patch.
- Parameters
-
id is the id of the patch dimension is the dimension of the patch origin is the origin of the domain length is the length of the domain dh is the maximum allowed mesh spacing
Definition at line 185 of file volcartesian.cpp.
◆ VolCartesian() [8/8]
bitpit::VolCartesian::VolCartesian | ( | std::istream & | stream | ) |
Creates a serial patch restoring the patch saved in the specified stream.
- Parameters
-
stream is the stream to read from
Definition at line 208 of file volcartesian.cpp.
Member Function Documentation
◆ _dump()
|
overrideprotectedvirtual |
Write the patch to the specified stream.
- Parameters
-
stream is the stream to write to
Implements bitpit::PatchKernel.
Definition at line 1085 of file volcartesian.cpp.
◆ _findCellEdgeNeighs()
|
overrideprotectedvirtual |
Extracts the neighbours of the specified cell for the given edge.
This function can be only used with three-dimensional cells.
- Parameters
-
id is the id of the cell edge is an edge of the cell blackList is a list of cells that are excluded from the search. The blacklist has to be a pointer to a unique list of ordered cell ids or a null pointer if no cells should be excluded from the search [in,out] neighs is the vector were the neighbours of the specified cell for the given edge will be stored. The vector is not cleared before adding the neighbours, it is extended by appending all the neighbours found by this function
Reimplemented from bitpit::PatchKernel.
Definition at line 1630 of file volcartesian.cpp.
◆ _findCellFaceNeighs()
|
overrideprotectedvirtual |
Extracts the neighbours of the specified cell for the given face.
- Parameters
-
id is the id of the cell face is a face of the cell blackList is a list of cells that are excluded from the search. The blacklist has to be a pointer to a unique list of ordered cell ids or a null pointer if no cells should be excluded from the search [in,out] neighs is the vector were the neighbours will be stored. The vector is not cleared before adding the neighbours, it is extended by appending all the neighbours found by this function
Reimplemented from bitpit::PatchKernel.
Definition at line 1603 of file volcartesian.cpp.
◆ _findCellVertexNeighs()
|
overrideprotectedvirtual |
Extracts the neighbours of the specified cell for the given local vertex.
- Parameters
-
id is the id of the cell vertex is a vertex of the cell blackList is a list of cells that are excluded from the search. The blacklist has to be a pointer to a unique list of ordered cell ids or a null pointer if no cells should be excluded from the search [in,out] neighs is the vector were the neighbours of the specified cell for the given vertex will be stored. The vector is not cleared before adding the neighbours, it is extended by appending all the neighbours found by this function
Reimplemented from bitpit::PatchKernel.
Definition at line 1665 of file volcartesian.cpp.
◆ _getDumpVersion()
|
overrideprotectedvirtual |
Get the version associated to the binary dumps.
- Returns
- The version associated to the binary dumps.
Implements bitpit::PatchKernel.
Definition at line 1073 of file volcartesian.cpp.
◆ _restore()
|
overrideprotectedvirtual |
Restore the patch from the specified stream.
- Parameters
-
stream is the stream to read from
Implements bitpit::PatchKernel.
Definition at line 1119 of file volcartesian.cpp.
◆ _updateAdjacencies()
|
overrideprotectedvirtual |
Internal function to update the adjacencies of the patch.
The function will always update the adjacencies of all the cells.
Reimplemented from bitpit::PatchKernel.
Definition at line 260 of file volcartesian.cpp.
◆ _updateInterfaces()
|
overrideprotectedvirtual |
Internal function to update the interfaces of the patch.
It is not possible to partially update the interfaces of this patch. The function will always update all the interfaces.
Reimplemented from bitpit::PatchKernel.
Definition at line 309 of file volcartesian.cpp.
◆ clone()
|
overridevirtual |
Creates a clone of the pach.
- Returns
- A clone of the pach.
Implements bitpit::PatchKernel.
Definition at line 224 of file volcartesian.cpp.
◆ convertToCellData()
std::vector< double > bitpit::VolCartesian::convertToCellData | ( | const std::vector< double > & | vertexData | ) | const |
Transform node data to cell data by calculating the mean of incident vertices of each cell
- Parameters
-
[in] vertexData contains the data on vertices
- Returns
- The vertex data converted to cell data.
Definition at line 1995 of file volcartesian.cpp.
◆ convertToVertexData()
std::vector< double > bitpit::VolCartesian::convertToVertexData | ( | const std::vector< double > & | cellData | ) | const |
Transform cell data to point data by calculating the mean of incident cells in each vertex.
- Parameters
-
[in] cellData contains the data on cells
- Returns
- The cell data converted to vertex data.
Definition at line 1940 of file volcartesian.cpp.
◆ evalCellBoundingBox()
|
overridevirtual |
Evaluates the bounding box of the specified cell.
- Parameters
-
id is the id of the cell [out] minPoint is the minimum point of the bounding box [out] maxPoint is the maximum point of the bounding box
Reimplemented from bitpit::PatchKernel.
Definition at line 867 of file volcartesian.cpp.
◆ evalCellCentroid() [1/2]
std::array< double, 3 > bitpit::VolCartesian::evalCellCentroid | ( | const std::array< int, 3 > & | ijk | ) | const |
Evaluates the centroid of the specified cell.
- Parameters
-
ijk is the set of cartesian indices of the cell
- Returns
- The centroid of the specified cell.
Definition at line 2232 of file volcartesian.cpp.
◆ evalCellCentroid() [2/2]
|
overridevirtual |
Evaluates the centroid of the specified cell.
- Parameters
-
id is the id of the cell
- Returns
- The centroid of the specified cell.
Reimplemented from bitpit::PatchKernel.
Definition at line 2219 of file volcartesian.cpp.
◆ evalCellSize() [1/2]
double bitpit::VolCartesian::evalCellSize | ( | const std::array< int, 3 > & | ijk | ) | const |
Evaluates the characteristic size of the specified cell.
- Parameters
-
ijk is the set of cartesian indices of the cell
- Returns
- The characteristic size of the specified cell.
Definition at line 841 of file volcartesian.cpp.
◆ evalCellSize() [2/2]
|
overridevirtual |
Evaluates the characteristic size of the specified cell.
- Parameters
-
id is the id of the cell
- Returns
- The characteristic size of the specified cell.
Implements bitpit::PatchKernel.
Definition at line 828 of file volcartesian.cpp.
◆ evalCellVolume() [1/2]
double bitpit::VolCartesian::evalCellVolume | ( | const std::array< int, 3 > & | ijk | ) | const |
Evaluates the volume of the specified cell.
- Parameters
-
ijk is the set of cartesian indices of the cell
- Returns
- The volume of the specified cell.
Definition at line 803 of file volcartesian.cpp.
◆ evalCellVolume() [2/2]
|
overridevirtual |
Evaluates the volume of the specified cell.
- Parameters
-
id is the id of the cell
- Returns
- The volume of the specified cell.
Implements bitpit::VolumeKernel.
Definition at line 790 of file volcartesian.cpp.
◆ evalInterfaceArea()
|
overridevirtual |
Evaluates the area of the specified interface.
- Parameters
-
id is the id of the interface
- Returns
- The area of the specified interface.
Implements bitpit::VolumeKernel.
Definition at line 882 of file volcartesian.cpp.
◆ evalInterfaceNormal()
|
overridevirtual |
Evaluates the normal of the specified interface.
- Parameters
-
id is the id of the interface
- Returns
- The normal of the specified interface.
Implements bitpit::VolumeKernel.
Definition at line 897 of file volcartesian.cpp.
◆ evalVertexCoords() [1/2]
std::array< double, 3 > bitpit::VolCartesian::evalVertexCoords | ( | const std::array< int, 3 > & | ijk | ) | const |
Evaluates the coordinate of the specified vertex.
- Parameters
-
ijk is the set of cartesian indices of the vertex
- Returns
- The coordinate of the specified vertex.
Definition at line 757 of file volcartesian.cpp.
◆ evalVertexCoords() [2/2]
std::array< double, 3 > bitpit::VolCartesian::evalVertexCoords | ( | long | id | ) | const |
Evaluates the coordinate of the specified vertex.
- Parameters
-
id is the id of the vertex
- Returns
- The coordinate of the specified vertex.
Definition at line 744 of file volcartesian.cpp.
◆ extractCellSubSet() [1/3]
std::vector< long > bitpit::VolCartesian::extractCellSubSet | ( | int | idMin, |
int | idMax ) const |
Extract a cell subset.
- Parameters
-
[in] idMin is the linear index of the lower bound [in] idMax is the linear index of the upper bound
- Returns
- The linear indices of the cell subset.
Definition at line 1724 of file volcartesian.cpp.
◆ extractCellSubSet() [2/3]
std::vector< long > bitpit::VolCartesian::extractCellSubSet | ( | std::array< double, 3 > const & | pointMin, |
std::array< double, 3 > const & | pointMax ) const |
Extract a cell subset.
- Parameters
-
[in] pointMin is the lower bound [in] pointMax is the upper bound
- Returns
- The linear indices of the cell subset.
Definition at line 1736 of file volcartesian.cpp.
◆ extractCellSubSet() [3/3]
std::vector< long > bitpit::VolCartesian::extractCellSubSet | ( | std::array< int, 3 > const & | ijkMin, |
std::array< int, 3 > const & | ijkMax ) const |
Extract a cell subset.
- Parameters
-
[in] ijkMin is the set of cartesian indices of the lower bound [in] ijkMax is the set of cartesian indices of the upper bound
- Returns
- The linear indices of the cell subset.
Definition at line 1695 of file volcartesian.cpp.
◆ extractVertexSubSet() [1/3]
std::vector< long > bitpit::VolCartesian::extractVertexSubSet | ( | int | idMin, |
int | idMax ) const |
Extract a vertex subset.
- Parameters
-
[in] idMin is the linear index of the lower bound [in] idMax is the linear index of the upper bound
- Returns
- The linear indices of the vertex subset.
Definition at line 1777 of file volcartesian.cpp.
◆ extractVertexSubSet() [2/3]
std::vector< long > bitpit::VolCartesian::extractVertexSubSet | ( | std::array< double, 3 > const & | pointMin, |
std::array< double, 3 > const & | pointMax ) const |
Extract a vertex subset.
- Parameters
-
[in] pointMin is the lower bound [in] pointMax is the upper bound
- Returns
- The linear indices of the vertex subset.
Definition at line 1789 of file volcartesian.cpp.
◆ extractVertexSubSet() [3/3]
std::vector< long > bitpit::VolCartesian::extractVertexSubSet | ( | std::array< int, 3 > const & | ijkMin, |
std::array< int, 3 > const & | ijkMax ) const |
Extract a vertex subset.
- Parameters
-
[in] ijkMin is the set of cartesian indices of the lower bound [in] ijkMax is the set of cartesian indices of the upper bound
- Returns
- The linear indices of the vertex subset.
Definition at line 1748 of file volcartesian.cpp.
◆ getCellCartesianId()
std::array< int, 3 > bitpit::VolCartesian::getCellCartesianId | ( | long | id | ) | const |
Converts a cell linear index to a set of cartesian indices.
The set of cartesian indices (i, j, k) identifies the position of a cell along the dimensions x, y, and z (in this specific order). If there are no cells along a direction, the corresponding cartesian index is set to a negative number.
No check on bounds is performed.
- Parameters
-
[in] id is the linear index of the cell
- Returns
- Returns the set of cartesian indices of the cell.
Definition at line 1402 of file volcartesian.cpp.
◆ getCellCentroids()
const std::vector< double > & bitpit::VolCartesian::getCellCentroids | ( | int | direction | ) | const |
Get cell centroids along the specified direction.
- Parameters
-
direction is the direction along which cell centroids are requested
- Returns
- Cell centroids along the specified direction.
Definition at line 2252 of file volcartesian.cpp.
◆ getCellCount() [1/2]
|
overridevirtual |
Gets the number of cells in the patch.
- Returns
- The number of cells in the patch
Reimplemented from bitpit::PatchKernel.
Definition at line 658 of file volcartesian.cpp.
◆ getCellCount() [2/2]
int bitpit::VolCartesian::getCellCount | ( | int | direction | ) | const |
Gets the number of cells along the specified direction.
- Parameters
-
direction is the direction along which cell count is requested
- Returns
- The number of cells along the specified.
Definition at line 669 of file volcartesian.cpp.
◆ getCellFaceNeighsCartesianId()
std::array< int, 3 > bitpit::VolCartesian::getCellFaceNeighsCartesianId | ( | long | id, |
int | face ) const |
Evaluate the cartesian index of the neighbour for the specified face.
- Parameters
-
id is the id of the cell face is a face of the cell
- Returns
- The cartesian index of the neighbour for the specified face.
Definition at line 2264 of file volcartesian.cpp.
◆ getCellFaceNeighsLinearId()
long bitpit::VolCartesian::getCellFaceNeighsLinearId | ( | long | id, |
int | face ) const |
Evaluate the linear index of the neighbour for the specified face.
- Parameters
-
id is the id of the cell face is a face of the cell
- Returns
- The linear index of the neighbour for the specified face.
Definition at line 2286 of file volcartesian.cpp.
◆ getCellLinearId() [1/2]
long bitpit::VolCartesian::getCellLinearId | ( | const std::array< int, 3 > & | ijk | ) | const |
Converts the cell cartesian notation to a linear notation.
The linear index is built incrementing first the cartesian index along direction x (index i), then the cartesian index along direction y (index j) and finally the cartesian index along direction z (index k). If there are no cells along a direction, that directon will not be considered for the generation of the linear index.
- Parameters
-
ijk is the set of cartesian indices of the cell
- Returns
- The linear id of the specified cell.
Definition at line 1368 of file volcartesian.cpp.
◆ getCellLinearId() [2/2]
long bitpit::VolCartesian::getCellLinearId | ( | int | i, |
int | j, | ||
int | k ) const |
Converts the cell cartesian notation to a linear notation.
The linear index is built incrementing first the cartesian index along direction x (index i), then the cartesian index along direction y (index j) and finally the cartesian index along direction z (index k). If there are no cells along a direction, that directon will not be considered for the generation of the linear index.
- Parameters
-
i is the cartesian index along the x direction j is the cartesian index along the y direction k is the cartesian index along the z direction
- Returns
- The linear id of the specified cell.
Definition at line 1351 of file volcartesian.cpp.
◆ getCellType() [1/2]
ElementType bitpit::VolCartesian::getCellType | ( | ) | const |
Gets the element type for the cells in the patch.
- Returns
- The element type for the cells in the patch.
Definition at line 692 of file volcartesian.cpp.
◆ getCellType() [2/2]
|
overridevirtual |
Gets the element type for the cell with the specified id.
- Parameters
-
id is the id of the requested cell
- Returns
- The element type for the cell with the specified id.
Reimplemented from bitpit::PatchKernel.
Definition at line 680 of file volcartesian.cpp.
◆ getInterfaceCount()
|
overridevirtual |
Gets the number of interfaces in the patch.
- Returns
- The number of interfaces in the patch
Reimplemented from bitpit::PatchKernel.
Definition at line 706 of file volcartesian.cpp.
◆ getInterfaceType() [1/2]
ElementType bitpit::VolCartesian::getInterfaceType | ( | ) | const |
Gets the element type for the interfaces in the patch.
- Returns
- The element type for the interfaces in the patch.
Definition at line 729 of file volcartesian.cpp.
◆ getInterfaceType() [2/2]
|
overridevirtual |
Gets the element type for the interface with the specified id.
- Parameters
-
id is the id of the requested interface
- Returns
- The element type for the interface with the specified id.
Reimplemented from bitpit::PatchKernel.
Definition at line 717 of file volcartesian.cpp.
◆ getLengths()
std::array< double, 3 > bitpit::VolCartesian::getLengths | ( | ) | const |
Gets the lengths of the sides of the box that defines the patch domain.
- Returns
- The lengths of the sides of the box that defines the patch domain.
Definition at line 1867 of file volcartesian.cpp.
◆ getMemoryMode()
VolCartesian::MemoryMode bitpit::VolCartesian::getMemoryMode | ( | ) | const |
Get the current memory mode.
- Returns
- The current memory mode.
Definition at line 986 of file volcartesian.cpp.
◆ getOrigin()
std::array< double, 3 > bitpit::VolCartesian::getOrigin | ( | ) | const |
Gets the origin of the patch.
The origin is the lower-left-back corner of the box that defines the patch domain.
- Returns
- The origin of the patch.
Definition at line 1802 of file volcartesian.cpp.
◆ getSpacing() [1/2]
std::array< double, 3 > bitpit::VolCartesian::getSpacing | ( | ) | const |
Get cell spacings of the patch.
- Returns
- Cell spacings of the patch.
Definition at line 910 of file volcartesian.cpp.
◆ getSpacing() [2/2]
double bitpit::VolCartesian::getSpacing | ( | int | direction | ) | const |
Get cell spacing along the specificed direction.
- Parameters
-
[in] direction is the direction along which the spacing is requested
- Returns
- The cell spacing along the specificed direction.
Definition at line 998 of file volcartesian.cpp.
◆ getVertexCartesianId() [1/3]
std::array< int, 3 > bitpit::VolCartesian::getVertexCartesianId | ( | const std::array< int, 3 > & | cellIjk, |
int | vertex ) const |
Gets the cartesian indices of the specified local vertex of a cell.
The set of cartesian indices (i, j, k) identifies the position of a vertex along the dimensions x, y, and z (in this specific order). If there are no cells along a direction, the corresponding cartesian index is set to a negative number.
No check on bounds is performed.
- Parameters
-
[in] cellIjk is the Cartesian cell index [in] vertex is the local vertex
- Returns
- Returns the set of cartesian indices of the vertex.
Definition at line 1563 of file volcartesian.cpp.
◆ getVertexCartesianId() [2/3]
std::array< int, 3 > bitpit::VolCartesian::getVertexCartesianId | ( | long | cellId, |
int | vertex ) const |
Gets the cartesian indices of the specified local vertex of a cell.
The set of cartesian indices (i, j, k) identifies the position of a vertex along the dimensions x, y, and z (in this specific order). If there are no cells along a direction, the corresponding cartesian index is set to a negative number.
No check on bounds is performed.
- Parameters
-
[in] cellId is the linear cell index [in] vertex is the local vertex
- Returns
- Returns the set of cartesian indices of the vertex.
Definition at line 1544 of file volcartesian.cpp.
◆ getVertexCartesianId() [3/3]
std::array< int, 3 > bitpit::VolCartesian::getVertexCartesianId | ( | long | id | ) | const |
Converts a vertex linear index to a set of cartesian indices.
The set of cartesian indices (i, j, k) identifies the position of a vertex along the dimensions x, y, and z (in this specific order). If there are no cells along a direction, the corresponding cartesian index is set to a negative number.
No check on bounds is performed.
- Parameters
-
[in] id is the linear index of the vertex
- Returns
- Returns the set of cartesian indices of the vertex.
Definition at line 1507 of file volcartesian.cpp.
◆ getVertexCoords()
const std::vector< double > & bitpit::VolCartesian::getVertexCoords | ( | int | direction | ) | const |
Get vertex coordinates along the specified direction.
- Parameters
-
direction is the direction along which vertex coordinates are requested
- Returns
- Vertex coordinates along the specified direction.
Definition at line 779 of file volcartesian.cpp.
◆ getVertexCount() [1/2]
|
overridevirtual |
Gets the number of vertices in the patch.
- Returns
- The number of vertices in the patch
Reimplemented from bitpit::PatchKernel.
Definition at line 637 of file volcartesian.cpp.
◆ getVertexCount() [2/2]
int bitpit::VolCartesian::getVertexCount | ( | int | direction | ) | const |
Gets the number of vertices along the specified direction.
- Parameters
-
direction is the direction along which vertex count is requested
- Returns
- The number of vertices along the specified.
Definition at line 648 of file volcartesian.cpp.
◆ getVertexLinearId() [1/2]
long bitpit::VolCartesian::getVertexLinearId | ( | const std::array< int, 3 > & | ijk | ) | const |
Converts the vertex cartesian notation to a linear notation.
The linear index is built incrementing first the cartesian index along direction x (index i), then the cartesian index along direction y (index j) and finally the cartesian index along direction z (index k). If there are no cells along a direction, that directon will not be considered for the generation of the linear index.
- Parameters
-
ijk is the set of cartesian indices of the vertex
- Returns
- The linear id of the specified vertex.
Definition at line 1473 of file volcartesian.cpp.
◆ getVertexLinearId() [2/2]
long bitpit::VolCartesian::getVertexLinearId | ( | int | i, |
int | j, | ||
int | k ) const |
Converts the vertex cartesian notation to a linear notation.
The linear index is built incrementing first the cartesian index along direction x (index i), then the cartesian index along direction y (index j) and finally the cartesian index along direction z (index k). If there are no cells along a direction, that directon will not be considered for the generation of the linear index.
- Parameters
-
i is the cartesian index along the x direction j is the cartesian index along the y direction k is the cartesian index along the z direction
- Returns
- The linear id of the specified vertex.
Definition at line 1456 of file volcartesian.cpp.
◆ isCellCartesianIdValid()
bool bitpit::VolCartesian::isCellCartesianIdValid | ( | const std::array< int, 3 > & | ijk | ) | const |
Checks if a cell cartesian index is valid.
- Parameters
-
ijk is the set of cartesian indices of the cell
- Returns
- Returns true if the index is valid, otherwise it returns false.
Definition at line 1431 of file volcartesian.cpp.
◆ isPointInside() [1/4]
|
overridevirtual |
Checks if the specified point is inside the patch.
- Parameters
-
[in] point is the point to be checked
- Returns
- Returns true if the point is inside the patch, false otherwise.
Implements bitpit::VolumeKernel.
Definition at line 1202 of file volcartesian.cpp.
◆ isPointInside() [2/4]
bool bitpit::VolumeKernel::isPointInside | ( | double | x, |
double | y, | ||
double | z ) const |
Checks if the specified point is inside the patch.
- Parameters
-
[in] x is the x coordinate of the point [in] y is the y coordinate of the point [in] z is the z coordinate of the point
- Returns
- Returns true if the point is inside the patch, false otherwise.
Definition at line 43 of file volume_kernel.cpp.
◆ isPointInside() [3/4]
|
overridevirtual |
Checks if the specified point is inside a cell.
- Parameters
-
[in] id is the idof the cell [in] point is the point to be checked
- Returns
- Returns true if the point is inside the cell, false otherwise.
Implements bitpit::VolumeKernel.
Definition at line 1176 of file volcartesian.cpp.
◆ isPointInside() [4/4]
bool bitpit::VolumeKernel::isPointInside | ( | long | id, |
double | x, | ||
double | y, | ||
double | z ) const |
Checks if the specified point is inside a cell.
- Parameters
-
[in] id is the index of the cells [in] x is the x coordinate of the point [in] y is the y coordinate of the point [in] z is the z coordinate of the point
- Returns
- Returns true if the point is inside the cell, false otherwise.
Definition at line 45 of file volume_kernel.cpp.
◆ isVertexCartesianIdValid()
bool bitpit::VolCartesian::isVertexCartesianIdValid | ( | const std::array< int, 3 > & | ijk | ) | const |
Checks if a vertex cartesian index is valid.
- Parameters
-
ijk is the set of cartesian indices of the vertex
- Returns
- Returns true if the index is valid, otherwise it returns false.
Definition at line 1580 of file volcartesian.cpp.
◆ linearCellInterpolation()
int bitpit::VolCartesian::linearCellInterpolation | ( | const std::array< double, 3 > & | point, |
std::vector< int > * | stencil, | ||
std::vector< double > * | weights ) const |
Calculates bi-/tri- linear interpolation stencil on cells for a given point.
At boundaries stencil is reduced to assure positive weights. If the point is outside a null-stencil is returned
- Parameters
-
[in] point are the point coordinates [out] stencil are the linear indices of the interpolation stencil [out] weights are the weights associated to stencil
- Returns
- The number of cells used in the interpolation stencil. If the point is outside a null stencil size is returned.
Definition at line 2053 of file volcartesian.cpp.
◆ linearVertexInterpolation()
int bitpit::VolCartesian::linearVertexInterpolation | ( | const std::array< double, 3 > & | point, |
std::vector< int > * | stencil, | ||
std::vector< double > * | weights ) const |
Calculates bi-/tri- linear interpolation stencil on vertex for a given point.
At boundaries stencil is reduced to assure positive weights. If the point is outside a null-stencil is returned
- Parameters
-
[in] point are the point coordinates [out] stencil are the linear indices of the interpolation stencil [out] weights are the weights associated to stencil
- Returns
- The number of cells used in the interpolation stencil. If the point is outside a null stencil size is returned.
Definition at line 2150 of file volcartesian.cpp.
◆ locateClosestCell()
long bitpit::VolCartesian::locateClosestCell | ( | std::array< double, 3 > const & | point | ) | const |
Locates the closest cell to point.
If the point is not inside the patch, the function returns the id of the cell the point projects on.
- Parameters
-
[in] point is the point to be checked
- Returns
- Returns the linear id of the closest cell to the point.
Definition at line 1309 of file volcartesian.cpp.
◆ locateClosestCellCartesian()
std::array< int, 3 > bitpit::VolCartesian::locateClosestCellCartesian | ( | std::array< double, 3 > const & | point | ) | const |
Locates the cell the closest cell to the point.
If the point is not inside the patch, the function returns the id of the cell the point projects on.
- Parameters
-
[in] point is the point to be checked
- Returns
- Returns the cartesian id of the closest cell to the point.
Definition at line 1324 of file volcartesian.cpp.
◆ locateClosestVertex()
long bitpit::VolCartesian::locateClosestVertex | ( | std::array< double, 3 > const & | point | ) | const |
Locates the closest vertex of the given point.
- Parameters
-
[in] point is the point
- Returns
- The linear id of the closest vertex of the given point.
Definition at line 1274 of file volcartesian.cpp.
◆ locateClosestVertexCartesian()
std::array< int, 3 > bitpit::VolCartesian::locateClosestVertexCartesian | ( | std::array< double, 3 > const & | point | ) | const |
Locates the closest vertex of the given point.
- Parameters
-
[in] point is the point
- Returns
- The set of Cartesian id of the closest vertex of the given point.
Definition at line 1286 of file volcartesian.cpp.
◆ locatePoint() [1/2]
|
overridevirtual |
Locates the cell the contains the point.
If the point is not inside the patch, the function returns the id of the null element.
- Parameters
-
[in] point is the point to be checked
- Returns
- Returns the linear id of the cell the contains the point. If the point is not inside the patch, the function returns the id of the null element.
Implements bitpit::PatchKernel.
Definition at line 1226 of file volcartesian.cpp.
◆ locatePoint() [2/2]
long bitpit::PatchKernel::locatePoint | ( | double | x, |
double | y, | ||
double | z ) const |
Locates the cell the contains the point.
If the point is not inside the patch, the function returns the id of the null element.
- Parameters
-
[in] x is the x coordinate of the point [in] y is the y coordinate of the point [in] z is the z coordinate of the point
- Returns
- Returns the id of the cell the contains the point. If the point is not inside the patch, the function returns the id of the null element.
Definition at line 646 of file patch_kernel.cpp.
◆ locatePointCartesian()
std::array< int, 3 > bitpit::VolCartesian::locatePointCartesian | ( | const std::array< double, 3 > & | point | ) | const |
Locates the cell the contains the point.
If the point is not inside the patch, the function returns the id of the null element.
- Parameters
-
[in] point is the point to be checked
- Returns
- Returns the set of cartesian id of the cell the contains the point. If the point is not inside the patch, the function returns negative ids.
Definition at line 1246 of file volcartesian.cpp.
◆ reset()
|
overridevirtual |
Function to reset the patch.
Reimplemented from bitpit::PatchKernel.
Definition at line 232 of file volcartesian.cpp.
◆ rotate()
|
overridevirtual |
Rotates the patch.
- Parameters
-
[in] n0 is a first point on the rotation axis [in] n1 is a second point on the rotation axis [in] angle is the rotation angle, expressed in radiants and positive for counterclockwise rotations
Reimplemented from bitpit::PatchKernel.
Definition at line 1853 of file volcartesian.cpp.
◆ scale()
|
overridevirtual |
Scales the patch.
- Parameters
-
[in] scaling is the scaling factor vector [in] center is the center of the scaling
Reimplemented from bitpit::PatchKernel.
Definition at line 1905 of file volcartesian.cpp.
◆ setLengths()
void bitpit::VolCartesian::setLengths | ( | const std::array< double, 3 > & | lengths | ) |
Sets the lengths of the sides of the box that defines the patch domain.
- Parameters
-
lengths is an array with the lengths of the sides of the box that defines the patch domain
Definition at line 1878 of file volcartesian.cpp.
◆ setOrigin()
void bitpit::VolCartesian::setOrigin | ( | const std::array< double, 3 > & | origin | ) |
Sets the origin of the patch.
The origin is the lower-left-back corner.
- Parameters
-
origin is the new origin of the patch
Definition at line 1814 of file volcartesian.cpp.
◆ switchMemoryMode()
void bitpit::VolCartesian::switchMemoryMode | ( | MemoryMode | mode | ) |
Switch to the specified memory mode.
- Parameters
-
mode is the memory mode that will be set
Definition at line 920 of file volcartesian.cpp.
◆ translate()
|
overridevirtual |
Translates the patch.
- Parameters
-
[in] translation is the translation vector
Reimplemented from bitpit::PatchKernel.
Definition at line 1825 of file volcartesian.cpp.
The documentation for this class was generated from the following files:
- src/volcartesian/volcartesian.hpp
- src/volcartesian/volcartesian.cpp
