VTKField handles geometry and data field information for the VTK format. More...
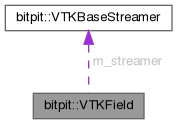
Public Member Functions | |
VTKField () | |
VTKField (const std::string &) | |
void | disable () |
void | enable () |
VTKFormat | getCodification () const |
unsigned | getComponentCount () const |
VTKDataType | getDataType () const |
VTKFieldType | getFieldType () const |
VTKLocation | getLocation () const |
const std::string & | getName () const |
uint64_t | getOffset () const |
std::fstream::pos_type | getPosition () const |
const VTKBaseStreamer & | getStreamer () const |
bool | hasAllMetaData () const |
bool | isEnabled () const |
void | read (std::fstream &, uint64_t, uint8_t) const |
void | setCodification (VTKFormat) |
void | setDataType (VTKDataType) |
void | setFieldType (VTKFieldType) |
void | setLocation (VTKLocation) |
void | setName (const std::string &) |
void | setOffset (uint64_t) |
void | setPosition (std::fstream::pos_type) |
void | setStreamer (VTKBaseStreamer &) |
void | write (std::fstream &) const |
Static Public Member Functions | |
static unsigned | getComponentCount (VTKFieldType fieldType) |
Protected Attributes | |
VTKFormat | m_codification |
VTKDataType | m_dataType |
bool | m_enabled |
VTKFieldType | m_fieldType |
VTKLocation | m_location |
std::string | m_name |
uint64_t | m_offset |
std::fstream::pos_type | m_position |
VTKBaseStreamer * | m_streamer |
Detailed Description
Constructor & Destructor Documentation
◆ VTKField() [1/2]
bitpit::VTKField::VTKField | ( | ) |
Default constructor
Definition at line 63 of file VTKField.cpp.
◆ VTKField() [2/2]
bitpit::VTKField::VTKField | ( | const std::string & | name | ) |
Member Function Documentation
◆ disable()
void bitpit::VTKField::disable | ( | ) |
Disables the field for writing/reading
Definition at line 159 of file VTKField.cpp.
◆ enable()
void bitpit::VTKField::enable | ( | ) |
Enables the field for writing/reading
Definition at line 152 of file VTKField.cpp.
◆ getCodification()
VTKFormat bitpit::VTKField::getCodification | ( | ) | const |
get codification of data field
- Returns
- codification [VTKFormat::APPENDED/VTKFormatASCII]
Definition at line 208 of file VTKField.cpp.
◆ getComponentCount() [1/2]
unsigned bitpit::VTKField::getComponentCount | ( | ) | const |
get the number of field components
- Returns
- number of field components
Definition at line 183 of file VTKField.cpp.
◆ getComponentCount() [2/2]
|
static |
get the number of field components
- Returns
- number of field components
Definition at line 40 of file VTKField.cpp.
◆ getDataType()
VTKDataType bitpit::VTKField::getDataType | ( | ) | const |
get type of data field
- Returns
- type of data field [ VTKDataType ]
Definition at line 192 of file VTKField.cpp.
◆ getFieldType()
VTKFieldType bitpit::VTKField::getFieldType | ( | ) | const |
get type of field
- Returns
- type of data field [ VTKFieldType ]
Definition at line 175 of file VTKField.cpp.
◆ getLocation()
VTKLocation bitpit::VTKField::getLocation | ( | ) | const |
get location of data field
- Returns
- location [VTKLocation::CELL/VTKLocationPOINT]
Definition at line 200 of file VTKField.cpp.
◆ getName()
const std::string & bitpit::VTKField::getName | ( | ) | const |
◆ getOffset()
uint64_t bitpit::VTKField::getOffset | ( | ) | const |
get offset in appended section
- Returns
- offset from "_" character in appended section
Definition at line 216 of file VTKField.cpp.
◆ getPosition()
std::fstream::pos_type bitpit::VTKField::getPosition | ( | ) | const |
get position of data field in VTK file. This information is available after VTK::ReadMetaData() has been called.
- Returns
- position in VTK file.
Definition at line 225 of file VTKField.cpp.
◆ getStreamer()
const VTKBaseStreamer & bitpit::VTKField::getStreamer | ( | ) | const |
get streamer of data field
- Returns
- streamer of data field
Definition at line 233 of file VTKField.cpp.
◆ hasAllMetaData()
bool bitpit::VTKField::hasAllMetaData | ( | ) | const |
Check if all information of field has been set
- Returns
- true if all information regarding field is available
Definition at line 249 of file VTKField.cpp.
◆ isEnabled()
bool bitpit::VTKField::isEnabled | ( | ) | const |
Returns if field is enabled for readind/writing
- Returns
- true if enabled
Definition at line 241 of file VTKField.cpp.
◆ read()
void bitpit::VTKField::read | ( | std::fstream & | str, |
uint64_t | entries, | ||
uint8_t | components ) const |
Reads the field through its streamer from file
- Parameters
-
[in] str file stream [in] entries total number of entries to be read [in] components size of subgroup
Definition at line 280 of file VTKField.cpp.
◆ setCodification()
void bitpit::VTKField::setCodification | ( | VTKFormat | cod | ) |
set codification of data field
- Parameters
-
[in] cod codification [VTKFormat::APPENDED/VTKFormatASCII]
Definition at line 113 of file VTKField.cpp.
◆ setDataType()
void bitpit::VTKField::setDataType | ( | VTKDataType | type | ) |
set type of data field
- Parameters
-
[in] type type of data [ VTKDataType::[[U]Int[8/16/32/64] / Float[32/64] ] ]
Definition at line 97 of file VTKField.cpp.
◆ setFieldType()
void bitpit::VTKField::setFieldType | ( | VTKFieldType | type | ) |
set type of data field
- Parameters
-
[in] type type of data field [ VTKFieldType::SCALAR/VECTOR/TENSOR ]
Definition at line 121 of file VTKField.cpp.
◆ setLocation()
void bitpit::VTKField::setLocation | ( | VTKLocation | loc | ) |
set location of data field
- Parameters
-
[in] loc location of data field [VTKLocation::CELL/VTKLocationPOINT]
Definition at line 105 of file VTKField.cpp.
◆ setName()
void bitpit::VTKField::setName | ( | const std::string & | name | ) |
set name of data field
- Parameters
-
[in] name name of data field
Definition at line 89 of file VTKField.cpp.
◆ setOffset()
void bitpit::VTKField::setOffset | ( | uint64_t | off | ) |
set offset of data field for appended output
- Parameters
-
[in] off offset from "_" character of appended section
Definition at line 137 of file VTKField.cpp.
◆ setPosition()
void bitpit::VTKField::setPosition | ( | std::fstream::pos_type | pos | ) |
set position of data field
- Parameters
-
[in] pos position of data field [VTKLocation::CELL/VTKLocationPOINT]
Definition at line 129 of file VTKField.cpp.
◆ setStreamer()
void bitpit::VTKField::setStreamer | ( | VTKBaseStreamer & | streamer | ) |
set streamer for reading and writing
- Parameters
-
[in] streamer streamer
Definition at line 145 of file VTKField.cpp.
◆ write()
void bitpit::VTKField::write | ( | std::fstream & | str | ) | const |
Writes the field through its streamer to file
- Parameters
-
[in] str file stream
Definition at line 268 of file VTKField.cpp.
Member Data Documentation
◆ m_codification
|
protected |
◆ m_dataType
|
protected |
type of data [ VTKDataType::[[U]Int[8/16/32/64] / Float[32/64] ]]
◆ m_enabled
|
protected |
◆ m_fieldType
|
protected |
◆ m_location
|
protected |
◆ m_name
|
protected |
◆ m_offset
|
protected |
◆ m_position
|
protected |
◆ m_streamer
|
protected |
The documentation for this class was generated from the following files:
- src/IO/VTK.hpp
- src/IO/VTKField.cpp
