Configuration file parser. More...
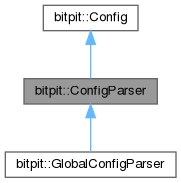
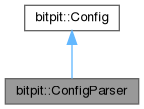
Public Member Functions | |
ConfigParser (const std::string &rootName) | |
ConfigParser (const std::string &rootName, bool multiSections) | |
ConfigParser (const std::string &rootName, int version) | |
ConfigParser (const std::string &rootName, int version, bool multiSections) | |
void | read (config::SourceFormat format, const std::string &content, bool append=true) |
void | read (const std::string &filename, bool append=true) |
void | reset (const std::string &rootName) |
void | reset (const std::string &rootName, int version) |
void | reset (const std::string &rootName, int version, bool multiSections) |
void | write (config::SourceFormat format, std::string *content) const |
void | write (const std::string &filename) const |
![]() | |
Config (bool multiSections=false) | |
Config (Config &&other)=default | |
Config (const Config &other) | |
Section & | addSection (const std::string &key) |
void | clear () |
void | dump (std::ostream &out, int indentLevel=0) const |
const std::string & | get (const std::string &key) const |
template<typename T> | |
T | get (const std::string &key) const |
std::string | get (const std::string &key, const std::string &fallback) const |
template<typename T> | |
T | get (const std::string &key, const T &fallback) const |
int | getOptionCount () const |
Options & | getOptions () |
const Options & | getOptions () const |
Section & | getSection (const std::string &key) |
const Section & | getSection (const std::string &key) const |
int | getSectionCount () const |
int | getSectionCount (const std::string &key) const |
Sections & | getSections () |
const Sections & | getSections () const |
MultiSection | getSections (const std::string &key) |
ConstMultiSection | getSections (const std::string &key) const |
bool | hasOption (const std::string &key) const |
bool | hasSection (const std::string &key) const |
bool | isMultiSectionsEnabled () const |
Config & | operator= (Config &&other)=default |
Config & | operator= (Config other) |
Section & | operator[] (const std::string &key) |
const Section & | operator[] (const std::string &key) const |
bool | removeOption (const std::string &key) |
bool | removeSection (const std::string &key) |
void | set (const std::string &key, const std::string &value) |
template<typename T> | |
void | set (const std::string &key, const T &value) |
void | swap (Config &other) |
Additional Inherited Members | |
![]() | |
typedef std::vector< const Section * > | ConstMultiSection |
typedef std::vector< Section * > | MultiSection |
typedef std::map< std::string, std::string > | Options |
typedef Config | Section |
typedef std::multimap< std::string, std::unique_ptr< Config > > | Sections |
![]() | |
bool | m_multiSections |
Detailed Description
Configuration file parser.
This class implements a configuration file parser.
Definition at line 38 of file configuration.hpp.
Constructor & Destructor Documentation
◆ ConfigParser() [1/4]
bitpit::ConfigParser::ConfigParser | ( | const std::string & | rootName | ) |
Construct a new configuration parser.
- Parameters
-
rootName is the name of the root element
Definition at line 54 of file configuration.cpp.
◆ ConfigParser() [2/4]
bitpit::ConfigParser::ConfigParser | ( | const std::string & | rootName, |
bool | multiSections ) |
Construct a new configuration parser.
- Parameters
-
rootName is the name of the root element multiSections if set to true the configuration parser will allow multiple sections with the same name
Definition at line 67 of file configuration.cpp.
◆ ConfigParser() [3/4]
bitpit::ConfigParser::ConfigParser | ( | const std::string & | rootName, |
int | version ) |
Construct a new configuration parser.
- Parameters
-
rootName is the name of the root element version is the required version
Definition at line 79 of file configuration.cpp.
◆ ConfigParser() [4/4]
bitpit::ConfigParser::ConfigParser | ( | const std::string & | rootName, |
int | version, | ||
bool | multiSections ) |
Construct a new configuration parser.
- Parameters
-
rootName is the name of the root element version is the required version multiSections if set to true the configuration parser will allow multiple sections with the same name
Definition at line 93 of file configuration.cpp.
Member Function Documentation
◆ read() [1/2]
void bitpit::ConfigParser::read | ( | config::SourceFormat | format, |
const std::string & | content, | ||
bool | append = true ) |
Read the specified content.
Configuration can be read from a content either in XML or JSON format.
- Parameters
-
format is the format of the content content is the content that will be read append controls if the configuration file will be appended to the current configuration or if the current configuration will be overwritten with the contents of the configuration file.
Definition at line 204 of file configuration.cpp.
◆ read() [2/2]
void bitpit::ConfigParser::read | ( | const std::string & | filename, |
bool | append = true ) |
Read the specified configuration file.
Configuration can be read either from XML or JSON files.
- Parameters
-
filename is the filename of the configuration file append controls if the configuration file will be appended to the current configuration or if the current configuration will be overwritten with the contents of the configuration file.
Definition at line 161 of file configuration.cpp.
◆ reset() [1/3]
void bitpit::ConfigParser::reset | ( | const std::string & | rootName | ) |
Resets the configuration parser.
MultiSection property set in construction is not affected.
- Parameters
-
rootName is the name of the root element
Definition at line 106 of file configuration.cpp.
◆ reset() [2/3]
void bitpit::ConfigParser::reset | ( | const std::string & | rootName, |
int | version ) |
Resets the configuration parser. MultiSections property set in construction is not affected.
- Parameters
-
rootName is the name of the root element version is the version
Definition at line 122 of file configuration.cpp.
◆ reset() [3/3]
void bitpit::ConfigParser::reset | ( | const std::string & | rootName, |
int | version, | ||
bool | multiSections ) |
Resets the configuration parser. MultiSections property set in construction is forced to the new value given by the parameter multiSection
- Parameters
-
rootName is the name of the root element version is the version multiSections if set to true the configuration parser will allow multiple sections with the same name
Definition at line 140 of file configuration.cpp.
◆ write() [1/2]
void bitpit::ConfigParser::write | ( | config::SourceFormat | format, |
std::string * | content ) const |
Write the configuration to the specified string.
Configuration can be written either in XML or JSON format.
- Parameters
-
format is the format that will be used to write the configuration content on output will contain the configuration in the specified format
Definition at line 259 of file configuration.cpp.
◆ write() [2/2]
void bitpit::ConfigParser::write | ( | const std::string & | filename | ) | const |
Write the configuration to the specified file.
Configuration can be written either to XML or JSON files.
- Parameters
-
filename is the filename where the configuration will be written to
Definition at line 229 of file configuration.cpp.
The documentation for this class was generated from the following files:
- src/IO/configuration.hpp
- src/IO/configuration.cpp
