utils_example_00005.cpp
124 mimmo::MimmoGeometry * mimmo3 = new mimmo::MimmoGeometry(mimmo::MimmoGeometry::IOMode::CONVERT);
void setWorkingOnTarget(bool)
Definition: ProjPatchOnSurface.cpp:150
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: ProjPrimitivesOnSurfaces.cpp:142
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setPatch(MimmoSharedPointer< MimmoObject > geo)
Definition: ProjPatchOnSurface.cpp:122
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface,...
Definition: ProjPatchOnSurface.hpp:99
MimmoSharedPointer< MimmoObject > getProjectedElement()
Definition: ProjPrimitivesOnSurfaces.cpp:129
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
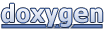