utils_example_00002.cpp
ControlDeformExtSurface is a class that check a deformation field, associated to a MimmoObject geomet...
Definition: ControlDeformExtSurface.hpp:105
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
@ POINT
void addConstraintFile(std::string file, int format)
Definition: ControlDeformExtSurface.cpp:254
void setDefField(dmpvecarr3E *field)
Definition: ControlDeformExtSurface.cpp:180
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void addConstraint(MimmoSharedPointer< MimmoObject > constraint)
Definition: ControlDeformExtSurface.cpp:220
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void setTolerance(double tol)
Definition: ControlDeformExtSurface.cpp:209
void setGeometry(MimmoSharedPointer< MimmoObject > target)
Definition: ControlDeformExtSurface.cpp:191
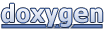