manipulators_example_00008.cpp
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
@ RANDOM
@ NONE
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setRandomSignature(uint32_t signature)
Definition: CreateSeedsOnSurface.cpp:328
void setRandomFixed(bool fix)
Definition: CreateSeedsOnSurface.cpp:316
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
Distribute a raw list of points on a target 3D surface.
Definition: CreateSeedsOnSurface.hpp:111
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setFilename(std::string filename)
Definition: GenericInput.cpp:131
void setEngineENUM(CSeedSurf)
Definition: CreateSeedsOnSurface.cpp:255
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
void setFunction(const MRBFBasisFunction &funct, bool isCompact=false)
Definition: MRBF.cpp:594
void setMassCenterAsSeed(bool)
Definition: CreateSeedsOnSurface.cpp:286
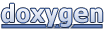