manipulators_example_00006.cpp
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWorkingOnTarget(bool)
Definition: ProjPatchOnSurface.cpp:150
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
@ NONE
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setInput(T *data)
GENERICINPUT////////////////////////////////////////////////////////////////////////////.
Definition: GenericInput.tpp:209
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface,...
Definition: ProjPatchOnSurface.hpp:99
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
CreatePointCloud manages cloud point data in raw format to create a MimmoObject Point Cloud container...
Definition: CreatePointCloud.hpp:91
void setFunction(const MRBFBasisFunction &funct, bool isCompact=false)
Definition: MRBF.cpp:594
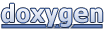