manipulators_example_00005.cpp
110 std::cout << " add pin info : " << std::boolalpha << mimmo::pin::addPin(mimmo0, lattice, M_GEOM, M_GEOM) << std::endl;
111 std::cout << " add pin info : " << std::boolalpha << mimmo::pin::addPin(input, lattice, M_DISPLS, M_DISPLS) << std::endl;
112 std::cout << " add pin info : " << std::boolalpha << mimmo::pin::addPin(lattice, applier, M_GDISPLS, M_GDISPLS) << std::endl;
113 std::cout << " add pin info : " << std::boolalpha << mimmo::pin::addPin(mimmo0, applier, M_GEOM, M_GEOM) << std::endl;
114 std::cout << " add pin info : " << std::boolalpha << mimmo::pin::addPin(applier, mimmo1, M_GEOM, M_GEOM) << std::endl;
@ CUBE
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setBuildSkdTree(bool build)
Definition: MimmoGeometry.cpp:466
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setOutputDebugResults(std::string path)
Definition: Chain.cpp:256
void setReadFromFile(bool readFromFile)
Definition: GenericInput.cpp:123
void setFilename(std::string filename)
Definition: GenericInput.cpp:131
void setLattice(darray3E &origin, darray3E &span, ShapeType, iarray3E &dimensions, iarray3E °rees)
Definition: FFDLattice.cpp:316
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
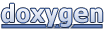