manipulators_example_00003.cpp
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicMeshes.cpp:619
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setFilename(std::string filename)
Definition: GenericOutput.cpp:134
int accessPointIndex(int i, int j, int k)
Definition: BasicMeshes.hpp:204
void setInput(T *data)
GENERICINPUT////////////////////////////////////////////////////////////////////////////.
Definition: GenericInput.tpp:209
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericOutput is the class that write generic data in a file output.
Definition: GenericOutput.hpp:109
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setReadFromFile(bool readFromFile)
Definition: GenericInput.cpp:123
void setLattice(darray3E &origin, darray3E &span, ShapeType, iarray3E &dimensions, iarray3E °rees)
Definition: FFDLattice.cpp:316
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
@ CYLINDER
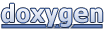