manipulators_example_00001.cpp
void setMaxDistance(double distance)
Definition: TwistGeometry.cpp:166
ScaleGeometry is the class that applies a scaling to a given geometry patch in respect to the mean po...
Definition: ScaleGeometry.hpp:78
void setDirection(darray3E direction)
Definition: TranslationGeometry.cpp:108
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
RotationGeometry is the class that applies a rotation to a given geometry patch.
Definition: RotationGeometry.hpp:79
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setDirection(darray3E direction)
Definition: TwistGeometry.cpp:139
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
BendGeometry applies custom bending deformations along axis-directions of a target geometry.
Definition: BendGeometry.hpp:120
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setTranslation(double alpha)
Definition: TranslationGeometry.cpp:119
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
void setRotation(double alpha)
Definition: RotationGeometry.cpp:146
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
TranslationGeometry is the class that applies a translation to a given geometry patch.
Definition: TranslationGeometry.hpp:75
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
TwistGeometry is the class that applies a twist to a given geometry patch.
Definition: TwistGeometry.hpp:85
void setDirection(darray3E direction)
Definition: RotationGeometry.cpp:135
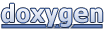