ioofoam_example_00002.cpp
57 mimmo::MimmoGeometry * surfacewriter = new mimmo::MimmoGeometry(mimmo::MimmoGeometry::IOMode::WRITE);
63 mimmo::MimmoGeometry * volumewriter = new mimmo::MimmoGeometry(mimmo::MimmoGeometry::IOMode::WRITE);
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
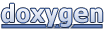