ioofoam_example_00001.cpp
@ CUBE
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setDisplacements(dvecarr3E displacements)
Definition: FFDLattice.cpp:284
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
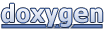