iocgns_example_00002.cpp
void setMinimumFaceValidityTolerance(double tol)
Definition: MeshChecker.cpp:235
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setMaximumVolumeTolerance(double tol)
Definition: MeshChecker.cpp:208
void setMaximumBoundarySkewnessTolerance(double tol)
Definition: MeshChecker.cpp:226
void setMinimumVolumeChangeTolerance(double tol)
Definition: MeshChecker.cpp:244
MeshChecker is the class to evaluate the quality of a volume mesh.
Definition: MeshChecker.hpp:87
void setMaximumSkewnessTolerance(double tol)
Definition: MeshChecker.cpp:217
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
void setMinimumVolumeTolerance(double tol)
Definition: MeshChecker.cpp:199
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
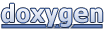