iocgns_example_00001.cpp
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
RotationGeometry is the class that applies a rotation to a given geometry patch.
Definition: RotationGeometry.hpp:79
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setRotation(double alpha)
Definition: RotationGeometry.cpp:146
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
Reconstruct a vector field from daughter mesh to mother mesh.
Definition: ReconstructFields.hpp:188
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setDirection(darray3E direction)
Definition: RotationGeometry.cpp:135
ExtractVectorField is specialized derived class of ExtractField to extract a vector field of array<do...
Definition: ExtractFields.hpp:263
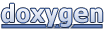