geohandlers_example_00004.cpp
99 mesh->addConnectedCell(livector1D({{0,2,3,1}}), bitpit::ElementType::QUAD, long(0), long(0), rank);
100 mesh->addConnectedCell(livector1D({{5,2,4,5,6,3}}), bitpit::ElementType::POLYGON, long(1), long(1), rank);
101 mesh->addConnectedCell(livector1D({{5,4,9,10,7,5}}), bitpit::ElementType::POLYGON, long(0), long(2), rank);
102 mesh->addConnectedCell(livector1D({{5,7,8,6}}), bitpit::ElementType::QUAD, long(1), long(3), rank);
103 mesh->addConnectedCell(livector1D({{7,10,11,8}}), bitpit::ElementType::QUAD, long(1), long(4), rank);
104 mesh->addConnectedCell(livector1D({{6,8,11}}), bitpit::ElementType::TRIANGLE, long(0), long(5), rank);
105 mesh->addConnectedCell(livector1D({{5,9,12,13,11,10}}), bitpit::ElementType::POLYGON, long(1), long(6), rank);
106 mesh->addConnectedCell(livector1D({{12,14,15,13}}), bitpit::ElementType::QUAD, long(0), long(7), rank);
107 mesh->addConnectedCell(livector1D({{14,16,17,15}}), bitpit::ElementType::QUAD, long(1), long(8), rank);
108 mesh->addConnectedCell(livector1D({{16,20,21,17}}), bitpit::ElementType::QUAD, long(0), long(9), rank);
109 mesh->addConnectedCell(livector1D({{6, 20,22, 23,24,25,21}}), bitpit::ElementType::POLYGON, long(1), long(10), rank);
110 mesh->addConnectedCell(livector1D({{22,26,27,23}}), bitpit::ElementType::QUAD, long(1), long(11), rank);
111 mesh->addConnectedCell(livector1D({{23,27,28,24}}), bitpit::ElementType::QUAD, long(0), long(12), rank);
112 mesh->addConnectedCell(livector1D({{24,28,29,25}}), bitpit::ElementType::QUAD, long(1), long(13), rank);
113 mesh->addConnectedCell(livector1D({{6,26,30,31,29,28,27}}), bitpit::ElementType::POLYGON, long(1), long(14), rank);
114 mesh->addConnectedCell(livector1D({{30,32,33,31}}), bitpit::ElementType::QUAD, long(0), long(15), rank);
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
ClipGeometry is a class that clip a 3D geometry according to a plane intersecting it.
Definition: ClipGeometry.hpp:85
Triangulate a target MimmoObject non-homogeneous and/or non-triangular surface mesh.
Definition: SurfaceTriangulator.hpp:74
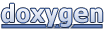