geohandlers_example_00003.cpp
void setTolerance(double tol=1.e-8)
Definition: SelectionByMapping.cpp:233
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
@ NONE
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
Selection mapping external surfaces/volume/3D curves on a target mesh of the same topology.
Definition: MeshSelection.hpp:458
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
Reconstruct a vector field from daughter mesh to mother mesh.
Definition: ReconstructFields.hpp:188
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
@ MAPPING
ExtractVectorField is specialized derived class of ExtractField to extract a vector field of array<do...
Definition: ExtractFields.hpp:263
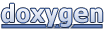