geohandlers_example_00000.cpp
void setInfLimits(double val, int dir)
Definition: BasicShapes.cpp:163
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicShapes.cpp:196
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
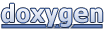