genericinput_example_00005.cpp
74 mimmo::IOWavefrontOBJ * mimmo1 = new mimmo::IOWavefrontOBJ(mimmo::IOWavefrontOBJ::IOMode::WRITE);
void setDir(const std::string &pathdir)
Definition: IOWavefrontOBJ.cpp:1384
void setTextureUVMode(bool UVmode)
Definition: IOWavefrontOBJ.cpp:1428
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
Executable block handling io of 3D surface polygonal mesh in *.obj format.
Definition: IOWavefrontOBJ.hpp:336
void setFilename(const std::string &name)
Definition: IOWavefrontOBJ.cpp:1393
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void printResumeFile(bool print)
Definition: IOWavefrontOBJ.cpp:1436
void setFunction(const MRBFBasisFunction &funct, bool isCompact=false)
Definition: MRBF.cpp:594
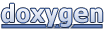