genericinput_example_00002.cpp
GenericOutputMPVData is the class that write a generic data to file output as mimmo::MimmoPiercedVect...
Definition: GenericOutput.hpp:203
void setFilename(std::string filename)
Definition: GenericOutput.cpp:326
GenericInputMPVData is the class that set a generic input data as mimmo::MimmoPiercedVector.
Definition: GenericInput.hpp:246
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setReadDir(std::string dir)
Definition: GenericInput.cpp:376
void setFilename(std::string filename)
Definition: GenericInput.cpp:368
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
void setWriteDir(std::string dir)
Definition: GenericOutput.cpp:317
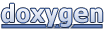