genericinput_example_00001.cpp
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setFilename(std::string filename)
Definition: GenericOutput.cpp:134
GenericOutput is the class that write generic data in a file output.
Definition: GenericOutput.hpp:109
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
void setReadFromFile(bool readFromFile)
Definition: GenericInput.cpp:123
void setFilename(std::string filename)
Definition: GenericInput.cpp:131
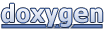