core_example_00001.cpp
90 mimmo0->getGeometry()->getPatch()->getVTK().addData("pointField", bitpit::VTKFieldType::SCALAR, bitpit::VTKLocation::POINT, field);
111 mimmo0->getGeometry()->getPatch()->getVTK().addData("cellField", bitpit::VTKFieldType::SCALAR, bitpit::VTKLocation::CELL, field);
132 mimmo0->getGeometry()->getPatch()->getVTK().addData("pointField", bitpit::VTKFieldType::SCALAR, bitpit::VTKLocation::POINT, field);
142 mimmo::MimmoPiercedVector<double> interfaceField = pointField.pointDataToBoundaryInterfaceData(p);
MimmoPiercedVector cellDataToPointData(double p=0.)
Definition: MimmoPiercedVector.tpp:682
MimmoPiercedVector pointDataToCellData(double p=0.)
Definition: MimmoPiercedVector.tpp:645
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
@ POINT
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
MimmoPiercedVector pointDataToBoundaryInterfaceData(double p=0.)
Definition: MimmoPiercedVector.tpp:785
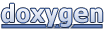