SkdTreeUtils.hpp
39 double distance(const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long &id, double r);
40 double signedDistance(const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long &id, std::array<double,3> &normal, double r);
41 void distance(int nP, const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long *id, double *distances, double r);
42 void distance(int nP, const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long *id, double *distances, double *r);
43 void signedDistance(int nP, const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long *ids, double *distances, std::array<double,3> *normals, double r);
44 void signedDistance(int nP, const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, long *ids, double *distances, std::array<double,3> *normals, double *r);
45 std::vector<long> selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol = 1.0e-04);
46 void extractTarget(bitpit::PatchSkdTree *target, const std::vector<const bitpit::SkdNode*> & leafSelection, std::vector<long> &extracted, double tol);
47 std::array<double,3> projectPoint(const std::array<double,3> *point, const bitpit::PatchSkdTree *tree, double r = std::numeric_limits<double>::max());
48 void projectPoint(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, std::array<double,3> *projected_points, long *ids, double r = std::numeric_limits<double>::max());
49 void projectPoint(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, std::array<double,3> *projected_points, long *ids, double* r);
52 double computePseudoNormal(const std::array<double,3> &point, const bitpit::SurfUnstructured *surface_mesh, long id, std::array<double, 3> & pseudo_normal);
54 bool checkPointBelongsToCell(const std::array<double, 3> &point, const bitpit::SurfUnstructured *surface_mesh, long id);
57 void globalDistance(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, double *distances, double r, bool shared = false);
58 void globalDistance(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, double *distances, double* r, bool shared = false);
59 void signedGlobalDistance(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, std::array<double,3> *normals, double *distances, double r, bool shared = false);
60 void signedGlobalDistance(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, std::array<double,3> *normals, double *distances, double* r, bool shared = false);
61 void projectPointGlobal(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, std::array<double,3> *projected_points, long *ids, int *ranks, double r = std::numeric_limits<double>::max(), bool shared = false);
62 void projectPointGlobal(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, std::array<double,3> *projected_points, long *ids, int *ranks, double* r, bool shared = false);
63 void locatePointOnGlobalPatch(int nP, const std::array<double,3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, bool shared = false);
64 std::vector<long> selectByGlobalPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol = 1.0e-04);
65 void extractTarget(bitpit::PatchSkdTree *target, const std::vector<bitpit::SkdBox> &leafSelectionBoxes, std::vector<long> &extracted, double tol);
66 long findSharedPointClosestGlobalCell(int nPoints, const std::array<double, 3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, double *distances, double r = std::numeric_limits<double>::max());
67 long findSharedPointClosestGlobalCell(int nPoints, const std::array<double, 3> *points, const bitpit::PatchSkdTree *tree, long *ids, int *ranks, double *distances, double *r);
71 long findPointClosestCell(const std::array<double,3> &point, const bitpit::PatchSkdTree *tree, bool interiorOnly, long *id, double *distance);
72 long findPointClosestCell(const std::array<double,3> &point, const bitpit::PatchSkdTree *tree, double maxDistance, bool interiorOnly, long *id, double *distance);
long findPointClosestCell(const std::array< double, 3 > &point, const bitpit::PatchSkdTree *tree, bool interiorOnly, long *id, double *distance)
Definition: SkdTreeUtils.cpp:1538
double signedDistance(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *tree, long &id, std::array< double, 3 > &normal, double r)
Definition: SkdTreeUtils.cpp:84
double computePseudoNormal(const std::array< double, 3 > &point, const bitpit::SurfUnstructured *surface_mesh, long id, std::array< double, 3 > &pseudo_normal)
Definition: SkdTreeUtils.cpp:517
bool checkPointBelongsToCell(const std::array< double, 3 > &point, const bitpit::SurfUnstructured *surface_mesh, long cellId)
Definition: SkdTreeUtils.cpp:592
void extractTarget(bitpit::PatchSkdTree *target, const std::vector< const bitpit::SkdNode * > &leafSelection, std::vector< long > &extracted, double tol)
Definition: SkdTreeUtils.cpp:296
long locatePointOnPatch(const std::array< double, 3 > &point, const bitpit::PatchSkdTree *tree)
Definition: SkdTreeUtils.cpp:456
std::vector< long > selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol)
Definition: SkdTreeUtils.cpp:251
darray3E projectPoint(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *skdtree, double r)
Definition: SkdTreeUtils.cpp:375
double distance(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *tree, long &id, double r)
Definition: SkdTreeUtils.cpp:49
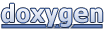